Sentiments Analysis of Financial News with News API and Transformers

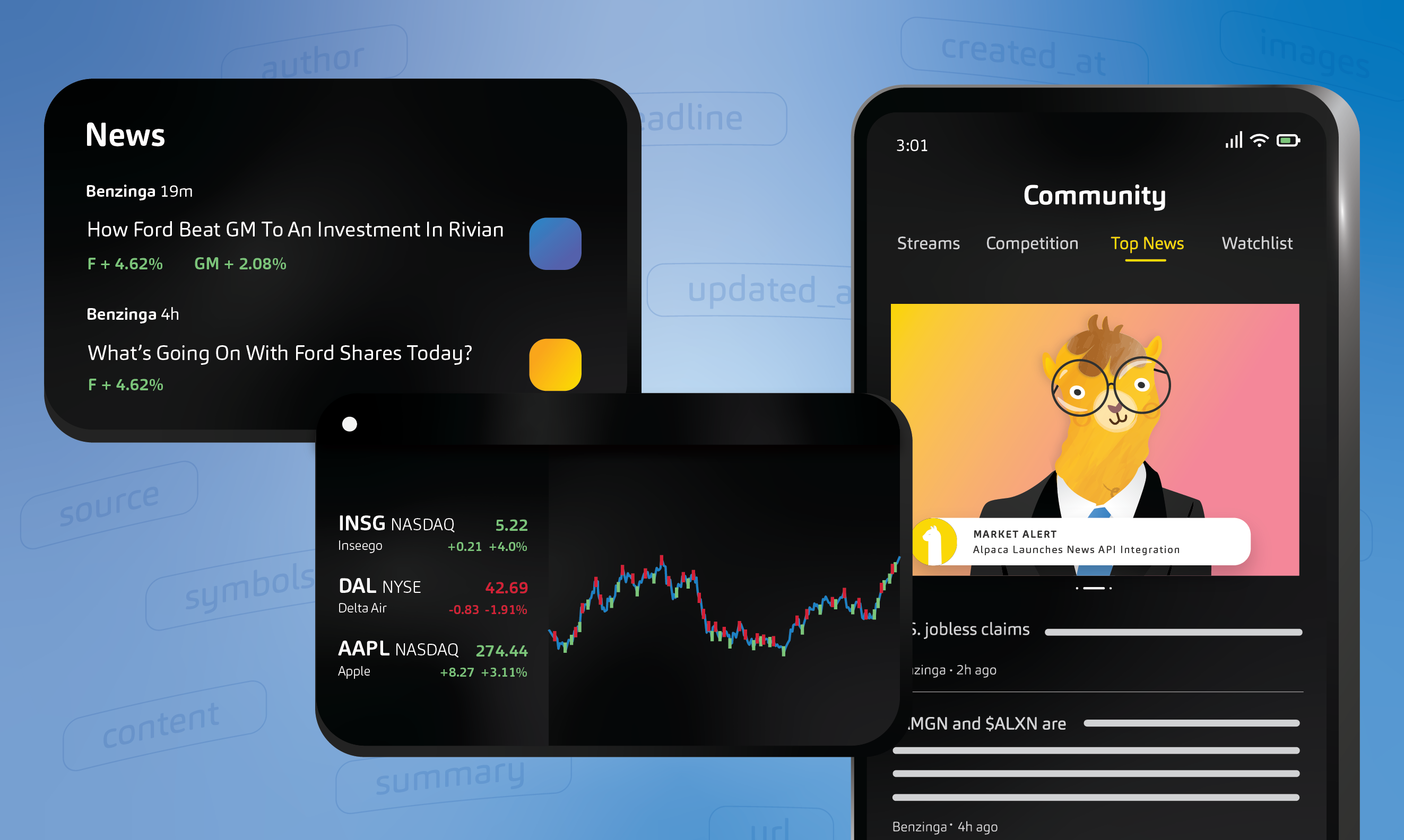
Alpaca now provides news data via API, allowing you to access market moving content from timely and trusted sources. 6+ years of historical news data can be accessed with the REST interface and live news data can be streamed with websockets. News data is available for over 1000 popular tickers across equities and cryptocurrencies.
Understanding a company’s news can be important in deciding whether one should invest in that company. Bad news can negatively impact a company’s stock price, and good news can have the opposite effect. In the following sections, we’ll learn how to use the news data API and Transformers, a natural language processing (NLP) library, to place trades based on sentiment analysis.
Getting Started with the News API
An easy way to access news data is through one of the language specific SDKs, available in Python, JavaScript, C#, and Go. We will be using the Python version as an example during this article. We can get started by first installing the SDK using pip.
pip install alpaca-trade-api
Now we can import alpaca_trade_api
and use our API keys to access news data.
from alpaca_trade_api import REST, Stream
API_KEY=’YOUR API KEY’
API_SECRET=’YOUR API SECRET’
Historical News Data
The rest_client
has the get_news
method which allows you to retrieve historical news data. It takes a few parameters that lets us specify the news data we want to query. For example, let’s say we want news data for AAPL
during 2021.
rest_client = REST(API_KEY, API_SECRET)
news = rest_client.get_news("AAPL", "2021-01-01", "2021-12-31")
The news data object that the method returns contains fields for each type of information, including the author, the headline, a summary of the data and the relevant symbols for that article. Look below to see an example of an article.
NewsV2({ 'author': 'Shanthi Rexaline',
'created_at': '2021-12-31T17:26:14Z',
'headline': 'This FAANG Stock Emerged As The Winner For 2021 -- And It '
"Isn't Apple",
'id': 24846669,
'images': [ { 'size': 'large',
'url': 'https://cdn.benzinga.com/files/imagecache/2048x1536xUP/images/story/2012/apples-4074608_1920.image_by_jamie_mahoney_from_pixabay.jpg'},
{ 'size': 'small',
'url': 'https://cdn.benzinga.com/files/imagecache/1024x768xUP/images/story/2012/apples-4074608_1920.image_by_jamie_mahoney_from_pixabay.jpg'},
{ 'size': 'thumb',
'url': 'https://cdn.benzinga.com/files/imagecache/250x187xUP/images/story/2012/apples-4074608_1920.image_by_jamie_mahoney_from_pixabay.jpg'}],
'source': '',
'summary': 'The FAANGs, which command a combined market capitalization of '
'a whopping $7.8 trillion, are five high-profile companies that '
'dominate the U.S. tech sector.',
'symbols': [ 'AAPL',
'AMD',
'AMZN',
'FB',
'GOOG',
'GOOGL',
'MSFT',
'NFLX',
'NVDA'],
'updated_at': '2021-12-31T17:35:03Z',
'url': 'https://www.benzinga.com/news/21/12/24846669/which-faang-stock-has-emerged-the-winner-for-2021-and-it-isnt-apple'}),
Note that if you are not using the SDK and requesting the REST API directly, the news data may sometimes be returned as raw HTML.
Live News Data
The websocket interface allows you to stream live news data. Creating a streaming client is similar to creating a REST client; we simply need to provide our API keys
stream_client = Stream(API_KEY, API_SECRET)
The streaming client works differently from the rest client. The streaming client requires us to provide a handler method which will receive the news data as it is published.
async def news_data_handler(news):
"""Will fire each time news data is published
"""
print(news)
stream_client.subscribe_news(news_data_handler, "AAPL")
stream_client.run()
Sentiment Analysis with Transformers
Sentiment analysis is the process of determining whether a piece of text provides a positive or negative attitude towards a subject. For example, we may want to determine whether some breaking news about a stock is good news or bad news. A simple way to do this can be counting the number of “good” words like “happy” or “strong” and the number of bad words such as “weak” or “low”.
But there are also more sophisticated methods involving machine learning models which are trained to classify text as positive or negative. In this example, we will use a pre-trained sentiment analysis model provided by the Transformers library. To get started, let’s first install Transformers.
pip install transformers
Now we can create a sentiment analysis model and then feed it text to analyze. The model will return whether it believes the text was positive or negative and the degree to which it is confident in its prediction. Here is an example from the Transformers documentation.
>>> from transformers import pipeline
# Allocate a pipeline for sentiment-analysis
>>> classifier = pipeline('sentiment-analysis')
>>> classifier('We are very happy to introduce pipeline to the transformers repository.')
[{'label': 'POSITIVE', 'score': 0.9996980428695679}]
Using Sentiment Analysis to Trade
In this section, we will use our sentiment analysis model to classify live news data as it is received from the news data API and then place trades via the trading API. In our news data handler, we can feed our model the news data as it arrives.
Sometimes certain fields in the news data may be empty, to make sure we look at all the relevant data available, we will perform sentiment analysis on the text data available in the headline and in the summary.
classifier = pipeline('sentiment-analysis')
async def news_data_handler(news):
"""Will fire each time news data is published
"""
summary = news.summary
headline = news.headline
relevant_text = summary + headline
sentiment = classifier(relevant_text)
if sentiment[‘label’] === ‘POSITIVE’ and sentiment[‘score’] > 0.95:
rest_client.submit_order(“AAPL”, 100)
elif sentiment[‘label’] === ‘NEGATIVE’ and sentiment[‘score’] > 0.95:
rest_client.submit_order(“AAPL”, -100)
stream_client.subscribe_news(news_data_handler, "AAPL")
stream_client.run()
Conclusion
The news data API opens up a new world of possibilities. We’ve seen how we can perform sentiment analysis on news data to determine whether we should go long or short a stock. But there are many other applications. For example, If you are building an OAuth or Broker API app, you can display news data to your users.
Please note that this article is for educational and informational purposes only. All screenshots are for illustrative purposes only. Alpaca does not recommend any specific securities or investment strategies.
Alpaca does not prepare, edit, or endorse Third Party Content. Alpaca does not guarantee the accuracy, timeliness, completeness or usefulness of Third Party Content, and is not responsible or liable for any content, advertising, products, or other materials on or available from third party sites.
Brokerage services are provided by Alpaca Securities LLC ("Alpaca"), member FINRA/SIPC, a wholly-owned subsidiary of AlpacaDB, Inc. Technology and services are offered by AlpacaDB, Inc.
This is not an offer, solicitation of an offer, or advice to buy or sell securities, or open a brokerage account in any jurisdiction where Alpaca is not registered (Alpaca is registered only in the United States).