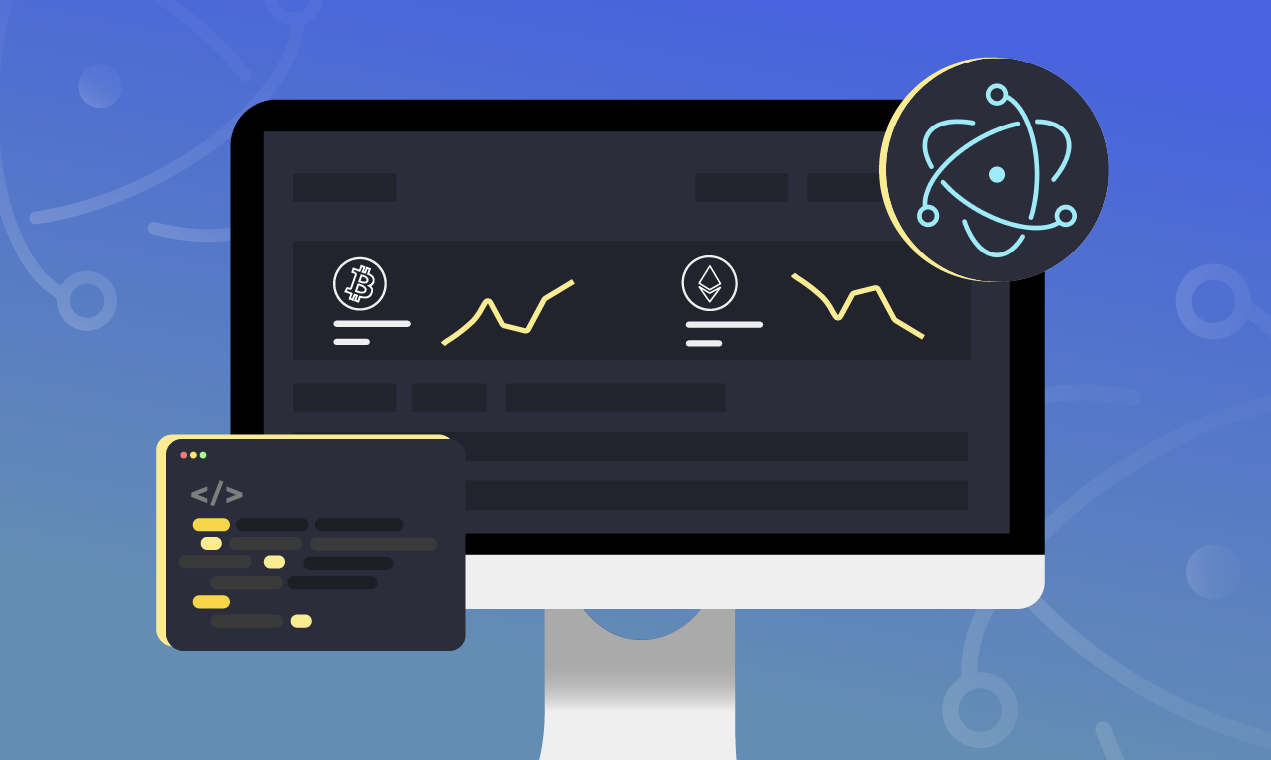
Introduction
Building desktop applications allows developers to leverage system APIs to implement extra features such as offline data storage, or sending scheduled notifications for users who have their browser windows closed.
For developers without experience in building native desktop applications using languages like Windows Form C#, the JavaScript ecosystem provides Electron.js.
What is Electron.js?
Electron.js is a JavaScript framework for building desktop applications across Windows, Linux, and Mac operating systems. Electron handles the underlying layer of your desktop application while you focus on building the application logic and user interface.
Electron.js offers developers a lower learning curve and shorter development time by using standard web technologies like HTML, JavaScript, and CSS to build desktop interfaces while exposing a set of powerful APIs.¹
Desktop applications built using Electron.js comprise one main process, alongside one or many renderer processes, which display the application interface. The renderer processes can also make use of frontend tools such as Vue.js and React for building interactive interfaces.
In the next section of this tutorial, you will build an electron application with two processes: one main and one renderer. The renderer process will display data on a single HTML web page.
An Electron App To Display Real Account Activities
At this point, you have some knowledge of Electron. Let’s proceed to get some hands-on experience of working with Electron by building a demo crypto application.
The demo application will retrieve and display all trading activities made on your Alpaca account from the Alpaca Trading API. To access the market API, this tutorial assumes that you have an Alpaca account and the Alpaca API keys. You will also need to install Node.js on your computer to run the Electron application.
Let’s get started with the first step of creating an Electron application.
Bootstrapping an Electron App
Open your computer’s terminal or command prompt to execute the commands in the following steps.
- Execute the command below to install the electron-generator package globally on your computer. The electron-generator package will help to bootstrap a boilerplate electron application with a single browser window.
The quick start guide of the electron documentation also contains steps on how to create an electron application from scratch.
npm install –global yo electron-generator
2. Execute the two commands below to create a directory named demo-alpaca-electron-bookmark and move into the directory. The new directory will contain the files for the electron application.
mkdir demo-alpaca-electron-bookmark
Next, execute the command below to generate an electron application using the installed electron-builder package.
yo electron
3. Execute the command below to run the electron application that was generated in the previous step.
yarn start
Modifying the Boilerplate Application
At this point, you have a boilerplate desktop application that displays text. In this section, you will proceed to modify the boilerplate application to display your Alpaca account activities.
- Open your computer terminal and execute the command below to install two additional dependencies into the electron application.
The dotenv package will retrieve your Alpaca secret credentials before the @alpacahq/alpaca-trade-api package establishes a connection with the Alpaca Trade API.
npm install @alpacahq/alpaca-trade-api package
2. Create a file named .env in the root directory of the electron application. The .env file contains your Alpaca API credentials for authentication.
Replace the placeholder values with the corresponding credentials and add the values into the .env file in the format below.
ALPACA_API_SECRET=API_SECRET
ALPACA_API_KEY=API_KEY
3. Using your preferred code editor, replace the content of the index.html file with the code below.
The code below modifies the description text within the default page and adds an unordered list (ul) element. The unordered list will display all your account activities retrieved from the /account/activities endpoint of the Trading API.
<!doctype html>
<html>
<head>
<meta charset="utf-8">
<title>demo alpaca electron bookmark</title>
<link rel="stylesheet" href="index.css">
</head>
<body>
<div class="container" style="padding: 1rem">
<nav>
<h1>Demo Alpaca Electron Bookmark</h1>
<p> A desktop application built to watch the price of an asset in your Toolbar</p>
</nav>
<hr/>
<section class="main" >
<h2> Account Activities </h2>
<ul id="activities-list"></ul>
</section>
<footer></footer>
</div>
</body>
</html>
4. To register a preload script for the browser window, open the index.js file and add the code highlighted below into the createMainWindow function.
The highlighted webPreference object contains a JavaScript file which we want to be executed immediately after the application is loaded.
const createMainWindow = async () => {
const win = new BrowserWindow({
title: app.name,
show: false,
width: 800,
height: 800,
webPreferences: {
preload: path.join(__dirname, "./application.js")
}
});
win.on('ready-to-show', () => {
win.show();
});
win.on('closed', () => {
// Dereference the window
// For multiple windows store them in an array
mainWindow = undefined;
});
await win.loadFile(path.join(__dirname, 'index.html'));
return win;
};
5. Next, create a JavaScript file named application.js. The application.js file will instantiate the Alpaca client class and retrieve your account activities.
The code below contains an event listener that uses the DOMContentLoaded event to detect when the HTML document for the index page has been loaded.
At the trigger of the event listener’s callback, the getAccountActivities method will be executed to retrieve an array containing 100 activities. The returning data array will be iterated upon, and a list containing the details of each activity will be added to the unordered list innerHTML property.
const Alpaca = require('@alpacahq/alpaca-trade-api')
const API_KEY = process.env.API_KEY
const API_SECRET = process.env.API_SECRET
const alpaca = new Alpaca({
keyId: API_KEY,
secretKey: API_SECRET,
paper: true,
})
window.addEventListener('DOMContentLoaded', async () => {
const ulElement = document.getElementById("activities-list")
const accountData = await alpaca.getAccountActivities({
until: new Date(),
pageSize: 100
})
if (accountData.length < 1) {
ulElement.innerHTML = `
<li>
<p> You have not performed any activity through your Alpaca account yet. </p>
</li>
`
return
}
accountData.map(({activity_type, symbol, transaction_time, cum_qty, side, price }, index) => {
ulElement.innerHTML += `
<li>
<p> ${cum_qty} ${side} order of ${symbol} on ${new Date(transaction_time).toLocaleDateString()} at ${price} </p>
</li>
`
})
})
Run the Modified Application
With the previous modifications, you can proceed to restart the electron processes to view the changes made to the application.
Press the ctrl + x key in the terminal running the electron application to stop it.
Next, execute the yarn start command to start the electron application once more.
At this point, you will find all the activities made on your Alpaca account displayed in the electron application.
Further Considerations
Congratulations on completing the steps in this tutorial! You now have hands-on experience of building cross-platform desktop applications using Electron.js. You can go through the @alpacahq/alpaca-trade-api documentation to learn more about the available methods.
If you want to leverage the steps within this tutorial as the foundation for building a more complex desktop application, we recommend that you consider the following;
Adding Offline Support
Desktop applications help make data available for offline use. In Electron applications, the HTML5 online and offline events can be used to detect the network connection status of the user’s computer. The Online/Offline Event Detection section of the electron documentation explains how to implement network detection.
Electron supports storing data in localstorage using community made packages such as electron-browser-storage. For complicated use cases, you can also use a lightweight NoSQL database such as NeDB to store data.
Encrypting Sensitive Data
Electron provides SafeStorage as part of its core API. SafeStorage encrypts a string before storing it locally on a computer. This is to prevent other programs from accessing the data.²
A valid use for SafeStorage will be when storing the user token returned back from the Alpaca OAuth flow.
Sources
- What is Electron? Electron JS.
- safeStorage. Electron JS.
Please note that this article is for general informational purposes only. All screenshots are for illustrative purposes only. Alpaca does not recommend any specific securities or investment strategies.
Alpaca does not prepare, edit, or endorse Third Party Content. Alpaca does not guarantee the accuracy, timeliness, completeness or usefulness of Third Party Content, and is not responsible or liable for any content, advertising, products, or other materials on or available from third party sites.
Cryptocurrency is highly speculative in nature, involves a high degree of risks, such as volatile market price swings, market manipulation, flash crashes, and cybersecurity risks. Cryptocurrency is not regulated or is lightly regulated in most countries. Cryptocurrency trading can lead to large, immediate and permanent loss of financial value. You should have appropriate knowledge and experience before engaging in cryptocurrency trading. For additional information please click here.
Cryptocurrency services are made available by Alpaca Crypto LLC ("Alpaca Crypto"), a FinCEN registered money services business (NMLS # 2160858), and a wholly-owned subsidiary of AlpacaDB, Inc. Alpaca Crypto is not a member of SIPC or FINRA. Cryptocurrencies are not stocks and your cryptocurrency investments are not protected by either FDIC or SIPC. Please see the Disclosure Library for more information.
This is not an offer, solicitation of an offer, or advice to buy or sell cryptocurrencies, or open a cryptocurrency account in any jurisdiction where Alpaca Crypto is not registered or licensed, as applicable.