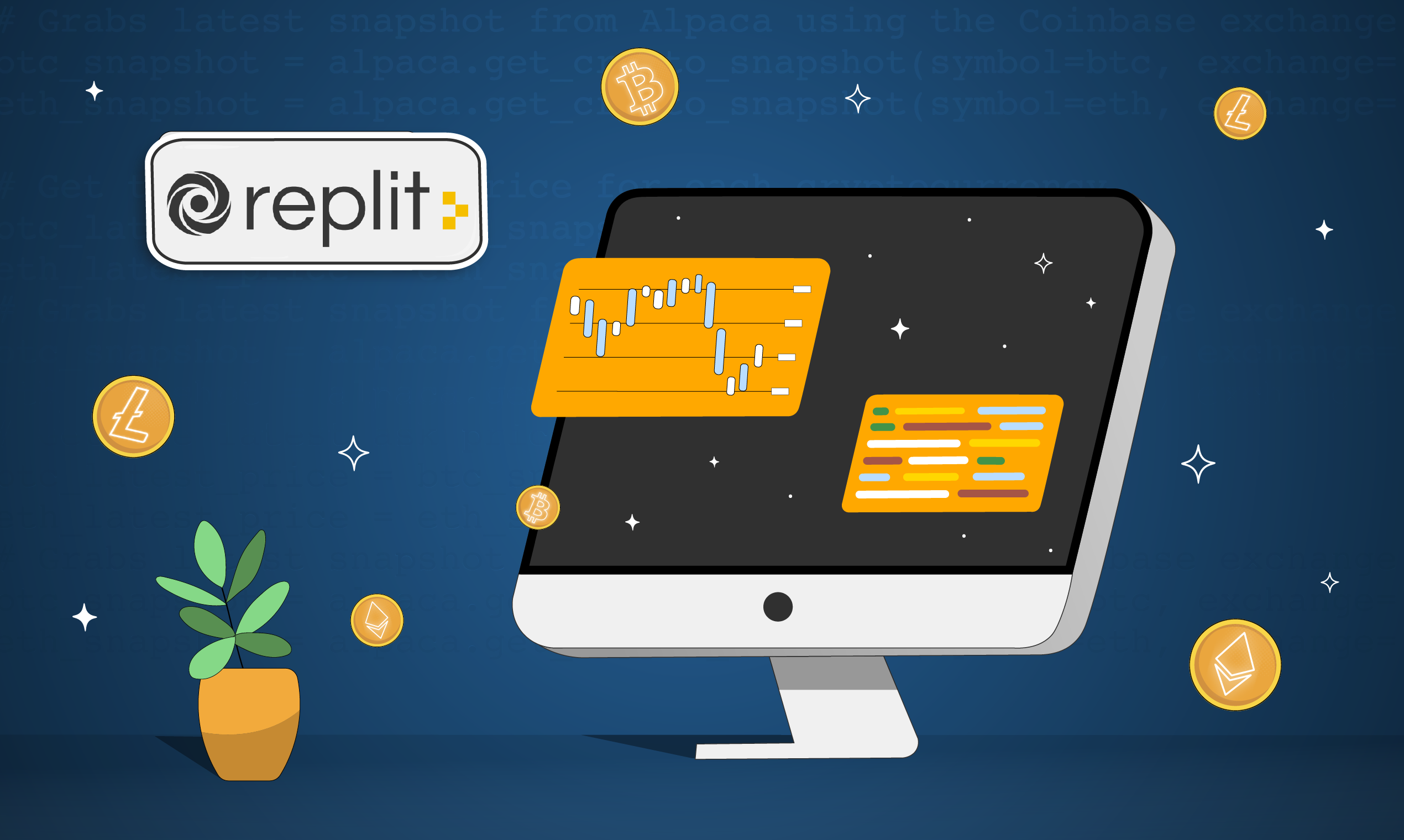
Blockchain technologies and cryptocurrencies have been taking off in popularity this year. With some speculative investors looking to get into the market, there is new demand[1] for a way to programmatically interact with crypto exchanges.
Alpaca provides easy-to-use APIs for trading crypto and requesting historical data, and in this article, we’ll walk you through a basic Repl that illustrates how you can do exactly that. We’re using Replit because it supplies a collaborative browser IDE that enables users to get started with projects quickly. This tutorial is just a starting point for one’s crypto journey with Alpaca and should give you the tools to start holding or coding your future strategies.
Getting started with Alpaca
To get started with Alpaca in Python, we’ll be using the Official Alpaca SDK on GitHub found here. We’ll be walking through how we can make simple HTTP requests using the provided client, and the first thing we need to do is locate our API keys. Your keys can be found by following this short guide.
For security reasons, it’s best to create environment variables for these keys when using Replit. Note that if you want to use this repl with a paper account, you’ll need to create an environment variable for the paper URL. The details on that can be found in the Alpaca SDK repo linked above. To access and create these variables, navigate to the padlock icon in the sidebar and assign your variables as key-value pairs.
Now that we have our API keys and we know how to set environment variables, we can access them as shown in the figure above. Next, we will declare the symbols of the cryptocurrencies we want to buy, in this case, we’ll be purchasing Bitcoin and Ethereum. Putting these steps together and importing the Alpaca SDK, we’re ready to start interacting with Alpaca’s endpoints.
# Import OS for environment variables (paper url and api credentials).
import os
import alpaca_trade_api as api
from math import floor
# Symbols for the cryptocurrencies we'd like to buy.
btc = "BTCUSD"
eth = "ETHUSD"
# Our API keys for Alpaca. Note if you're using a paper account,
# you'll need to insert the paper base URL as an env variable as well.
API_KEY = os.environ['API_KEY']
API_SECRET = os.environ['API_SECRET']
Getting your account data and crypto snapshots
Our next goal is to grab our account’s value, divide our portfolio evenly across Bitcoin and Ethereum, and get a market snapshot of their performance through the Coinbase exchange. The first thing you’ll need to do is create an instance of the Alpaca API using your account credentials.
# Setup an instance of the Alpaca API using our credentials.
alpaca = api.REST(API_KEY, API_SECRET)
This section accesses two endpoints: GET /v2/account
and GET /v1beta1/crypto/{symbol}/quotes
. Thankfully, the Alpaca SDK provides tools to do all the heavy lifting for you, and all you need to do is call methods on your instantiated object. All the methods and their responses are detailed in the documentation, and in our case, we’ll use get_account()
. After getting our account, we can access the cash property, cast it to a float, and divide it evenly.
# Getting our account's cash and splitting the available cash.
account = alpaca.get_account()
account_cash = float(account.cash)
cash_to_spend = account_cash / 2
The most recent crypto snapshots can be requested through the get_crypto_snapshot()
method. Since we’re looking to buy crypto at this price, we’ll grab the ask price from the latest quote from each snapshot’s properties.
# Grabs latest snapshot from Alpaca using the Coinbase exchange.
btc_snapshot = alpaca.get_crypto_snapshot(symbol=btc, exchange="CBSE")
eth_snapshot = alpaca.get_crypto_snapshot(symbol=eth, exchange="CBSE")
# Get the latest ask price for each cryptocurrency,
btc_latest_price = btc_snapshot.latest_quote.ap
eth_latest_price = eth_snapshot.latest_quote.ap
Figuring out how much to buy and submitting an order
This repl explores two different ways to submit orders for crypto: market orders and limit orders. The first one we’ll talk about is limit orders, where you’ll need to specify exactly how many units you want and the most you’d be willing to spend for each unit.
Armed with the knowledge of our available cash and the current ask price we can do some simple arithmetic to calculate the number of units to order. The division of cash by current price will yield a floating point number with more precision than what is allowed for Alpaca’s minimum order size, so we’ll round it off to four decimal places.
# This function will calculate the number of units one can afford given cash to spend and latest price, and round it down according to order of the precision factor.
def calculate_order_size(cash_to_spend, latest_price):
precision_factor = 10000
units_to_buy = floor(cash_to_spend * precision_factor / latest_price)
units_to_buy /= precision_factor
return units_to_buy
btc_units = calculate_order_size(cash_to_spend, btc_latest_price)
eth_units = calculate_order_size(cash_to_spend, eth_latest_price)
The provided method in the SDK for submitting an order is submit_order()
, so once we figure out our parameters we can place the order. The first parameter we’ll deal with is optional, the order type. Assigning this string accordingly, the other parameters of our order are the symbol, limit price, and quantity. All of these have been captured in variables earlier in the program, so we’ll simply reuse those.
# Now that we know how many to buy and for how much, we can place the orders.
order_type = "limit"
alpaca.submit_order(symbol=btc, qty=btc_units, type=order_type, limit_price=btc_latest_price)
alpaca.submit_order(symbol=eth, qty=eth_units, type=order_type, limit_price=eth_latest_price)
Submitting market orders is more straightforward than submitting limit orders. In essence, this type of order will let the market decide how many units you can afford given a set amount of cash. You’ll have less control over your order but it should fill immediately. Using the submit_order()
method again, the only parameters we need to specify this time are the symbol we’re buying and the notional value of the position you’d like to open up.
alpaca.submit_order(symbol=btc, notional=cash_to_spend)
alpaca.submit_order(symbol=eth, notional=cash_to_spend)
And that’s all there is to it! All four orders will have been placed and will soon be filled.
Conclusion
In this tutorial, we showed how the Alpaca Python SDK can be used to buy cryptocurrency at its current value. The SDK provides several methods that enable and simplify your market interactions as a whole, and hopefully, this tutorial has given you the tools to build upon these ideas.
Some next steps could be something as simple as adding more symbols to the project and refactoring the repeated code or even analyzing an SMA crossover strategy.
References
[1] J. Caporal, “Study: Over 50 million Americans likely to buy crypto in the next year: The ascent,” The Motley Fool, 17-Dec-2021. [Online]. Available: https://www.fool.com/the-ascent/research/study-americans-cryptocurrency/. [Accessed: 28-Dec-2021].
[2] “Secrets and environment variables,” Replit Docs. [Online]. Available: https://docs.replit.com/programming-ide/storing-sensitive-information-environment-variables. [Accessed: 07-Jan-2022].
Please note that this article is for informational purposes only. The example above is for illustrative purposes only. Actual crypto prices may vary depending on the market price at that particular time. Alpaca Crypto LLC does not recommend any specific cryptocurrencies.
Cryptocurrency is highly speculative in nature, involves a high degree of risks, such as volatile market price swings, market manipulation, flash crashes, and cybersecurity risks. Cryptocurrency is not regulated or is lightly regulated in most countries. Cryptocurrency trading can lead to large, immediate and permanent loss of financial value. You should have appropriate knowledge and experience before engaging in cryptocurrency trading. For additional information please click here.
Cryptocurrency services are made available by Alpaca Crypto LLC ("Alpaca Crypto"), a FinCEN registered money services business (NMLS # 2160858), and a wholly-owned subsidiary of AlpacaDB, Inc. Alpaca Crypto is not a member of SIPC or FINRA. Cryptocurrencies are not stocks and your cryptocurrency investments are not protected by either FDIC or SIPC. Please see the Disclosure Library for more information.
This is not an offer, solicitation of an offer, or advice to buy or sell cryptocurrencies, or open a cryptocurrency account in any jurisdiction where Alpaca Crypto is not registered or licensed, as applicable.