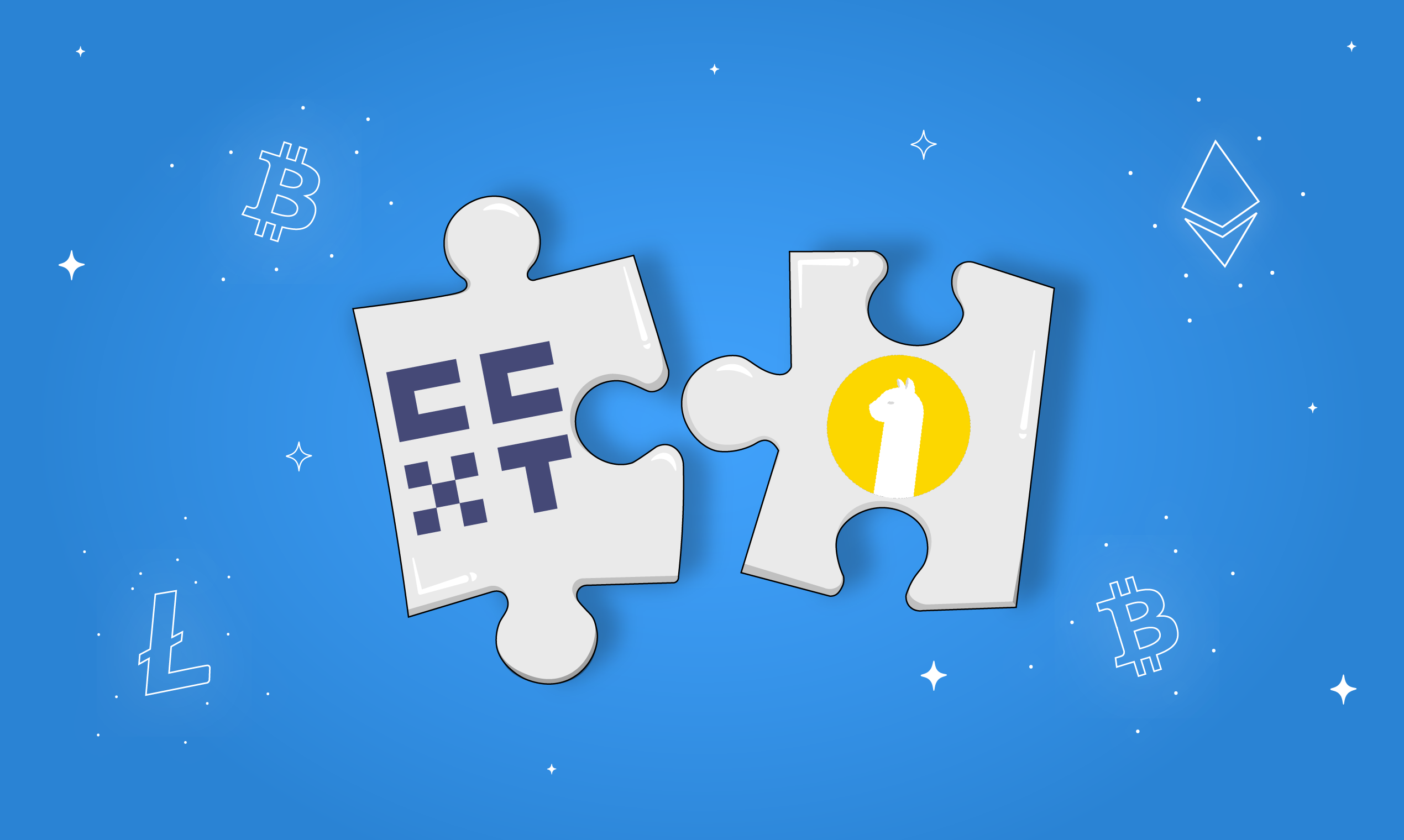
CCXT (CryptoCurrency eXchange Trading) is an open source library that lets you connect to dozens of cryptocurrency brokerages. These brokerages can be very different from each other, with different asset offerings and API services, often times making it difficult to transition from one brokerage to another. CCXT aims to make the transition easier by implementing the same interface across all brokerages on its platform. It does this by implementing a set of methods (e.g. createOrder, cancelOrder, etc.) for each brokerage by using the broker’s API endpoints to fulfill the requirements of the method.
Getting Started with CCXT
Installation
Use the following command to install CCXT:
pip install ccxt
Connecting Your Alpaca Brokerage Account
To start using your Alpaca brokerage account with CCXT, create an instance of the Alpaca exchange and supply it with your API keys.
import ccxt
API_KEY = "YOUR_ALPACA_API_KEY"
API_SECRET = "YOUR ALPACA_API_SECRET"
alpaca = ccxt.alpaca(({
apiKey: API_KEY ,
secret: API_SECRET,
})
# If we want to use paper api keys, enable sandbox mode
alpaca.set_sandbox_mode(True);
Accessing Data
Fetch Markets
Use the fetch_markets()
method to retrieve all tradable crypto and their properties.
markets = alpaca.fetch_markets()
Fetch Trades
Retrieve the latest trades using fetch_trades()
. You can supply a starting timestamp and a data limit to control the data you retrieve. If the since parameter is left out, you will receive the latest trades, and if the limit parameter is left out, there will be no cap on the number of trades received.
trades = alpaca.fetch_trades("BTCUSD", since=1637657082, limit=50)
Fetch OHLCV
Similarly, retrieve OHLCV (Bar) data with fetch_ohlcv
. This method will return the open, high, low, close, and volume data for the given ticker as a list of lists.
bars = alpaca.fetch_ohlcv("BTCUSD")
Creating and Managing Orders
Creating a New Order
CCXT’s method for creating a new order is very similar to Alpaca’s method. To submit an order, you will need to provide a symbol, order type, order side, quantity, and price if the order type is ‘limit’. The method will return details about the order, including its order id, which you will need if you want to manage the order.
order = alpaca.create_order("BTCUSD", "market", "buy", 0.01)
Canceling an Order
Use an existing order’s id to submit a request to cancel that order. Bear in mind that it isn't always possible to cancel an order. If the order is already filled, or if there is already a cancel request pending, the cancel request will not be successful.
order_id = order.id;
alpaca.cancel_order(order_id);
Accessing Open Orders
When managing orders, it’s helpful to know details about your open orders. This way you can easily cancel certain orders.
open_orders = alpaca.fetch_open_orders()
Conclusion
CCXT offers a great way to access Alpaca’s APIs. You can use it to transition between Alpaca and other crypto trading providers.
This article is for educational and informational purposes only. All screenshots are for illustrative purposes only.
Cryptocurrency is highly speculative in nature, involves a high degree of risks, such as volatile market price swings, market manipulation, flash crashes, and cybersecurity risks. Cryptocurrency is not regulated or is lightly regulated in most countries. Cryptocurrency trading can lead to large, immediate and permanent loss of financial value. You should have appropriate knowledge and experience before engaging in cryptocurrency trading. For additional information please click here.
Securities brokerage services are provided by Alpaca Securities LLC ("Alpaca Securities"), member FINRA/SIPC, a wholly-owned subsidiary of AlpacaDB, Inc. Technology and services are offered by AlpacaDB, Inc.
Cryptocurrency services are provided by Alpaca Crypto LLC ("Alpaca Crypto"), a wholly-owned subsidiary of AlpacaDB, Inc. Alpaca Crypto is not a member of SIPC or FINRA. Cryptocurrencies are not stocks and your cryptocurrency investments are not protected by either FDIC or SIPC.
This is not an offer, solicitation of an offer, or advice to buy or sell securities or cryptocurrencies, or open a brokerage account or cryptocurrency account in any jurisdiction where Alpaca Securities or Alpaca Crypto respectively, are not registered.