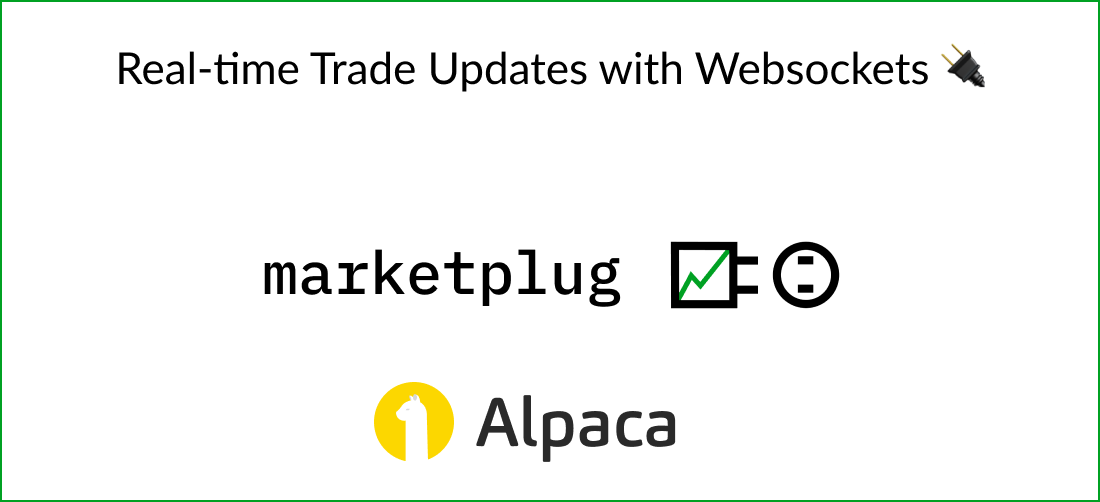
NOTE: This piece is broken up into three sections.
1). What is Marketplug? 2). How to sign up for Marketplug and link an Alpaca brokerage account? 3). How to work with Alpaca Websockets?What is Marketplug?
The idea for Marketplug came during the market meltdown in the weeks following COVID-19’s arrival. For weeks prior, I was obsessively following the wallstreetbets subreddit looking for trades that might help soften the blow of what was to come.

For those unfamiliar with wallstreetbets, it’s a very popular reddit trading forum for… adventurous traders. Posts were popping up left and right showing insane gains, (or losses). Things got so crazy, Bloomberg wrote a cover story it.
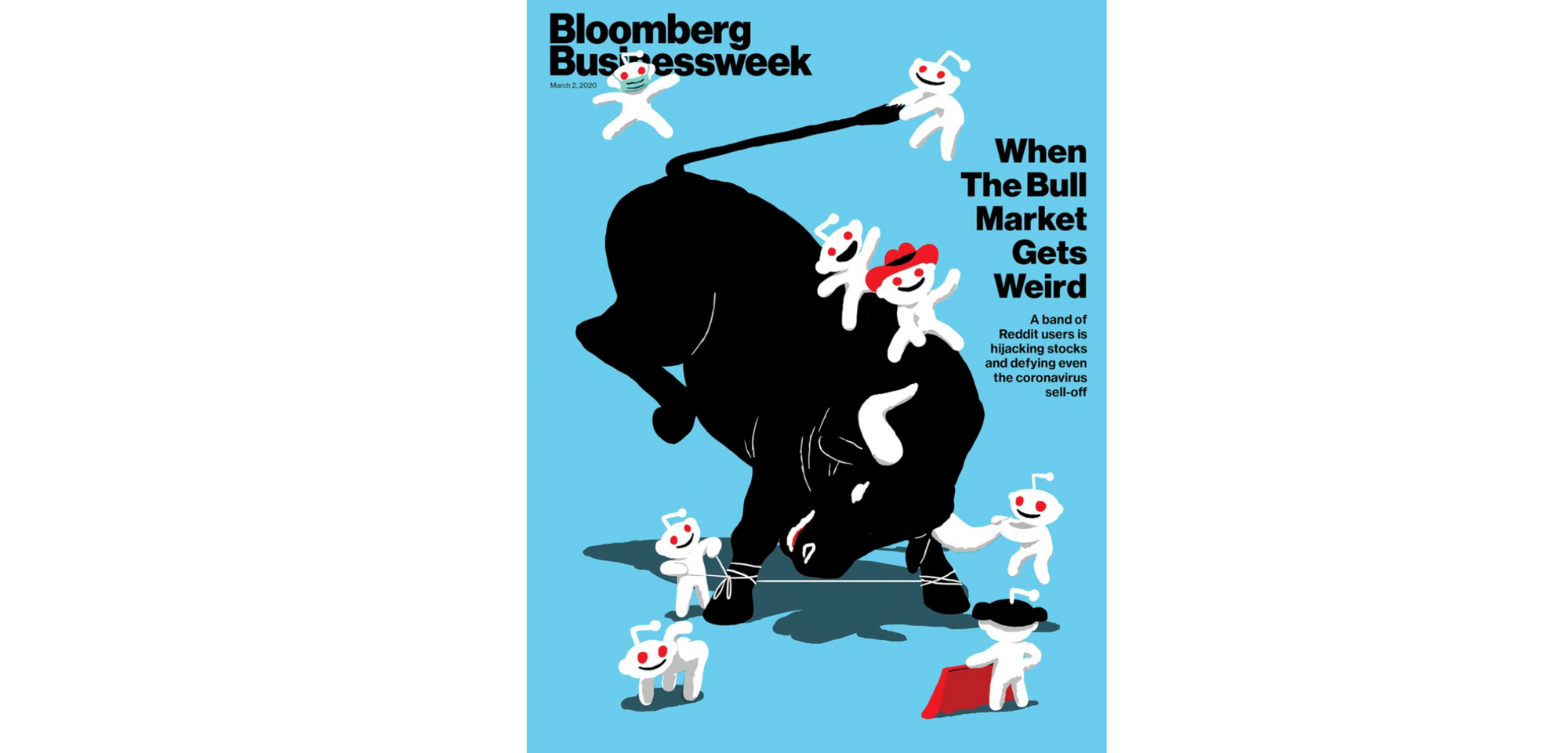
There was a ton of valuable information being exchanged — but identifying smart picks got a lot harder as the sub grew to more than a million members. Looking for new ideas and tips became like searching for a needle in a haystack. The endless scrolling through low-effort posts put me in an existential frame of mind. I wondered — how could trades be shared instantly? Why do I have to crawl through a ton of garbage, only to get a tip that looks like it might be coming from someone legit? Turns out, you can avoid all of this. That’s what Marketplug is for.
The idea behind Marketplug is to help investors find successful investors, follow them, and get their trades instantly. With API access to user accounts, users can see verified trading performance of other users.
Making this possible has been made a lot easier using the Alpaca API. Alpaca is an API built specifically for stock trading. Alpaca users can use the API to build trade algorithms, bots, and their own apps. Any Alpaca user can ‘plug’ their account into Marketplug, right now.

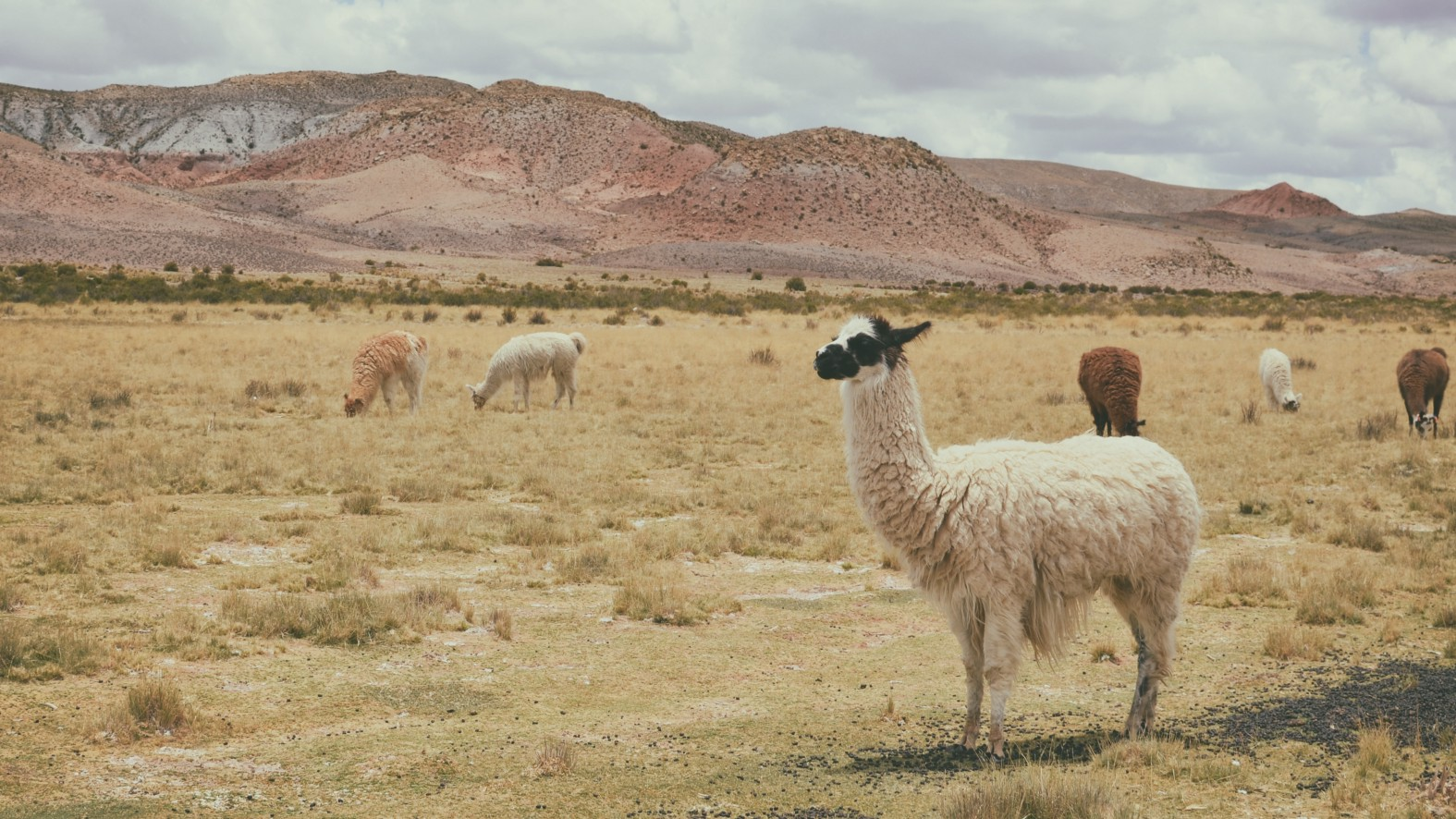
Once an account is ‘plugged’, the overall performance of that account is calculated, and users with the highest performance go up on the Marketplug ‘Top Performers’ leaderboard. Anytime a ‘plugged’ user makes a trade, their followers receive a text message with the details of that trade (minus the amount of money spent). Followers can then determine for themselves what to do with that information. This is a lot more direct than sifting through hundreds of reddit comments, or reading dozens of articles from spammy websites. If you just want some solid ideas to explore, you can follow users who’ve shown the ability to come up with solid ideas.
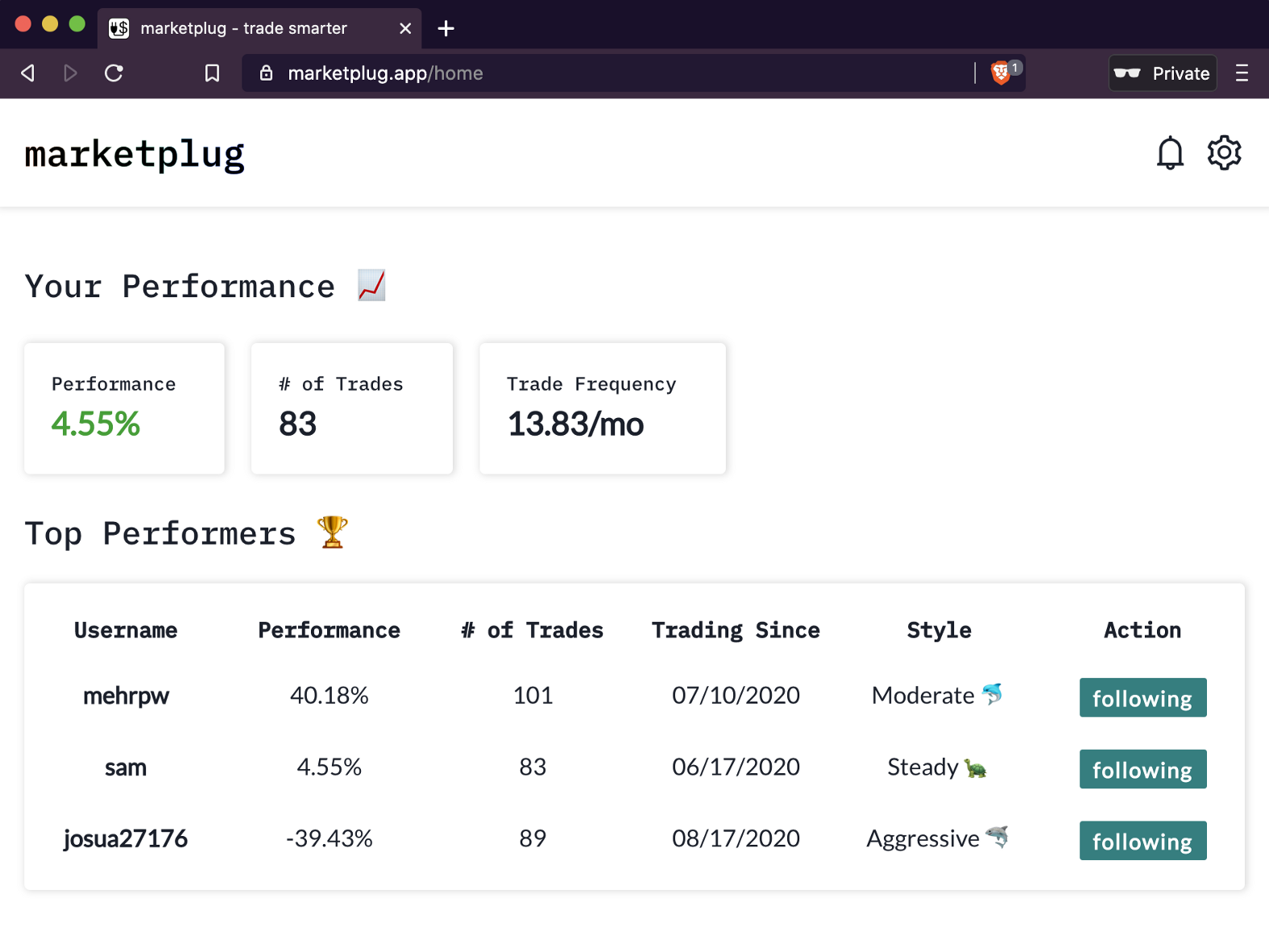
In addition to Alpaca, Marketplug also supports TD Ameritrade/ToS users. Users with one or both Alpaca or TD can plug any combination of their trading accounts into Marketplug. Support for more brokerages is in the works.
From here on, this post gets more technical. I’ll do a walkthrough of connecting an Alpaca account to Marketplug (easy), and outline some of the features. Finally, for those interested in technical/code details, I’ll do a walkthrough of a basic Alpaca /stream
Websockets implementation to get trade data, live from a user’s account.
How to sign up for Marketplug and link an Alpaca brokerage account?
Signing Up with Marketplug
The signup process is simple. First, go to the Marketplug website. In the top right corner, click ‘login or signup’, then click signup, and fill out the dropdown form. This first step registers you as a Marketplug user.
Once you’ve signed up as a Marketplug user you’ll see the ‘Connect Your Trading Accounts’ section.
Signing Up with Alpaca
In the ‘Connect Your Trading Accounts’ section, click ‘connect Alpaca’ — this will take you to Alpaca’s OAuth page, where you either log in, or click ‘Allow’. At this point, Marketplug is doing a couple of things:
- Authenticating your Alpaca account and retrieving your Auth token
- Fetching your Alpaca account data
- Calculating the Performance of your Alpaca account
- Fetching the past trades on your account
- Opening a websocket to your account, to listen for new trades
- Sending your account information to the database, from which your Dashboard and Profile are created
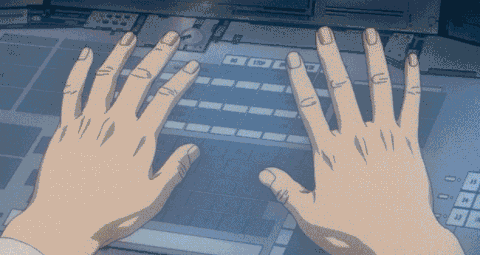
All of this is done on Marketplug’s side, with React and Node. There are some great tutorials that Alpaca’s user’s have written that cover points 1–4 ( check out ‘Alpaca Oauth, React, and Firebase’ <- web, and ‘How to Build a Fintech Investing App w/ Alpaca API’ <- iOS, both linked below).
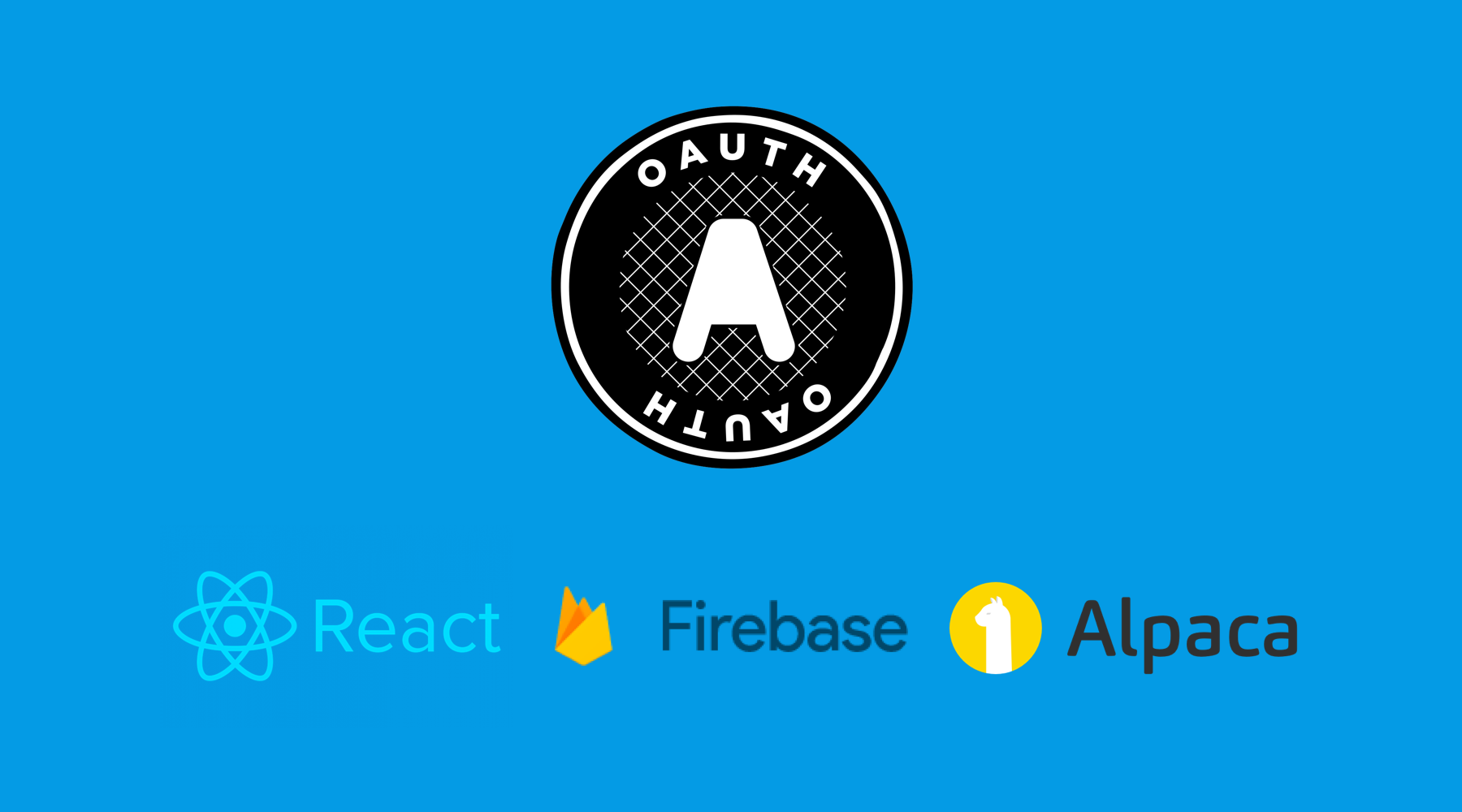
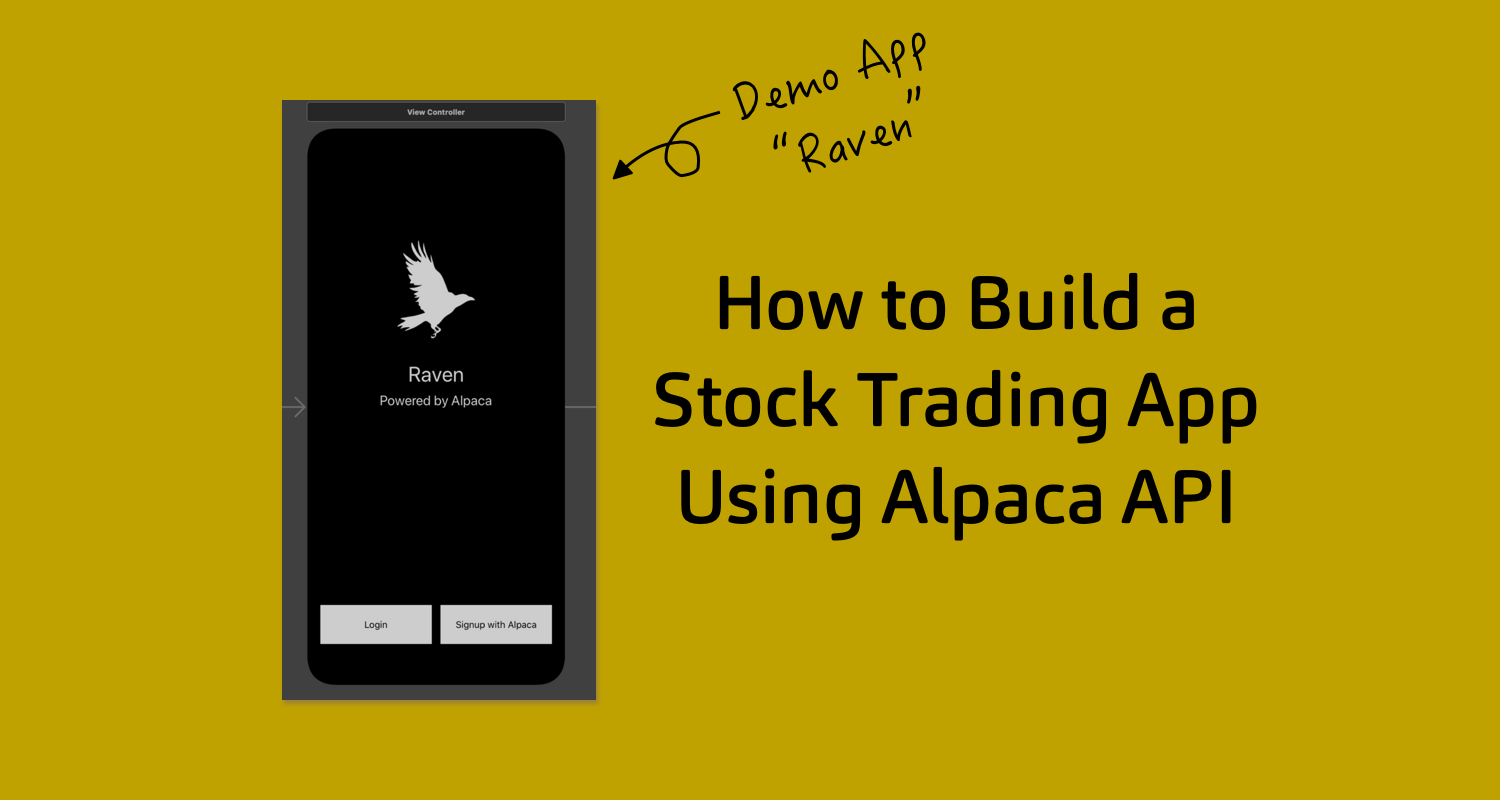
How to work with Alpaca Websockets?
Before I start, in case you’re unfamiliar with Websockets, here’s how I like to think of them. Most data on websites or apps, is served by a request to a data source. I think of it as a phone call. The site ‘calls’ the data source, and says “give me the price of AAPL stock”. If all goes well, the data source sends back something like “$124.98”, then hangs up.
A Websocket is like an open phone line. In this case, the site ‘calls’ and says “give me the price of AAPL stock, anytime it changes. I’ll stay on the line”. Since stock prices change often, the response(s) go something like “$124.98… $124.99… $125.01…”, and so on, until the Websocket is closed. That is an extremely non-technical explanation. You can get more of the nitty-gritty technical details here: MDN Websockets
OK, let’s start actually coding.
The following assumes that you have a Node server up and running, and have gone through the OAuth process, retrieving and storing a user’s access_token
.
Websocket management can be a bit tricky, luckily there are good libraries that provide utilities for dealing with common Websocket configuration and issues.
For a solid, standard, Websocket wrapper — use the ws library (docs).
A library used for handling disconnected Websockets is aptly named reconnecting-websocket (docs).
To start, add ws and the reconnecting-websocket libraries to your project, in the terminal:
npm install --save ws reconnecting-websocket
Import the libraries into your file, and set up your Alpaca /stream
endpoint (using the Paper account endpoint in this example). Lastly, make sure to grab the access_token
from you’re Alpaca login function:
// alpaca-api.js
// Import websocket libraries
const Websocket = require('ws');
const ReconnectingWebSocket = require('reconnecting-websocket');
// set up the Alpaca stream endpoint
const ALPACA_STREAM_URL = "wss://paper-api.alpaca.markets/stream";
// get the access_token from your OAuth Login function
const { access_token } = loginAlpaca();
There are 3 basic steps to opening a websocket that subscribes to a User’s Trades for Alpaca’s /stream
endpoint:
- Open a Websocket
- Send a message to authenticate to Alpaca’s streams (using
access_token)
- Send a message to ‘subscribe’ to Alpaca’s
trade_updates
stream
// alpaca-api.js
// Import Libraries and set up Stream endpoint here (Step 1) ✅
// 1. Define a function for Websocket Instantiation
const alpacaOpenStream = () => {
const options = {
WebSocket,
maxRetries: 100,
};
// this creates the Reconnecting Websocket, it will be passed in proceeding steps
const ws = new ReconnectingWebSocket(ALPACA_STREAM_URL, [], options);
return ws;
};
// 2. Define a function for Alpaca /stream Authentication
const alpacaSubscribeAuth = async (ws, access_token) => {
ws.send(
JSON.stringify({
action: 'authenticate',
data: {
oauth_token: access_token,
},
})
);
};
// 3. Define a function for subscribing to the Alpaca 'trade_updates' stream
const alpacaSubscribeToTrades = (ws) => {
ws.send(
JSON.stringify({
action: 'listen',
data: {
streams: ['trade_updates'],
},
})
);
};
With those functions set up, it’s time to set up a parent function for creating the websocket, and setting up listeners, for incoming messages. This function is what you will ultimately call to get the stream running:
const createSocket = (access_token) => {
// websocket setup
const ws = alpacaOpenStream();
ws.addEventListener('open', async function open() {
// authenticate, then subscribe to trade_updates
await alpacaSubscribeAuth(ws, access_token);
alpacaSubscribeToTrades(ws);
console.log('Opened ⚡️tream ⚡️ocket');
});
ws.addEventListener('message', function incoming(msg) {
console.log('Message: ? ', JSON.parse(msg.data));
if (msg.stream === 'trade_updates' && msg.data.event === 'new') {
console.log('New Trade ?: ', msg.data);
}
});
return ws;
};
// finally call the function!
createSocket(access_token);
As you can see above, you first create the Websocket ( const ws =
) — then you add event listeners ( ws.addEventListener(...)
) for open
and message
events. The trade_updates
stream will send messages anytime trade events happen, which your event listener will pick up. In the code above, if the trade msg
is new
— the console will log it.
That’s all folks — for a full copy of the code above, check out this Gist link. For more details on the Alpaca /stream
endpoint, check out this link to the official Alpaca docs.

Hope this was helpful and informative. Don’t forget to check out Marketplug, use Alpaca to build trading bots or apps of your own, the world is yours!

If you have any questions, comments, or just want to talk, feel free to shoot me a message at [email protected] or @marketplugapp on Twitter.
Technology and services are offered by AlpacaDB, Inc. Brokerage services are provided by Alpaca Securities LLC (alpaca.markets), member FINRA/SIPC. Alpaca Securities LLC is a wholly-owned subsidiary of AlpacaDB, Inc.
You can find us @AlpacaHQ, if you use twitter.