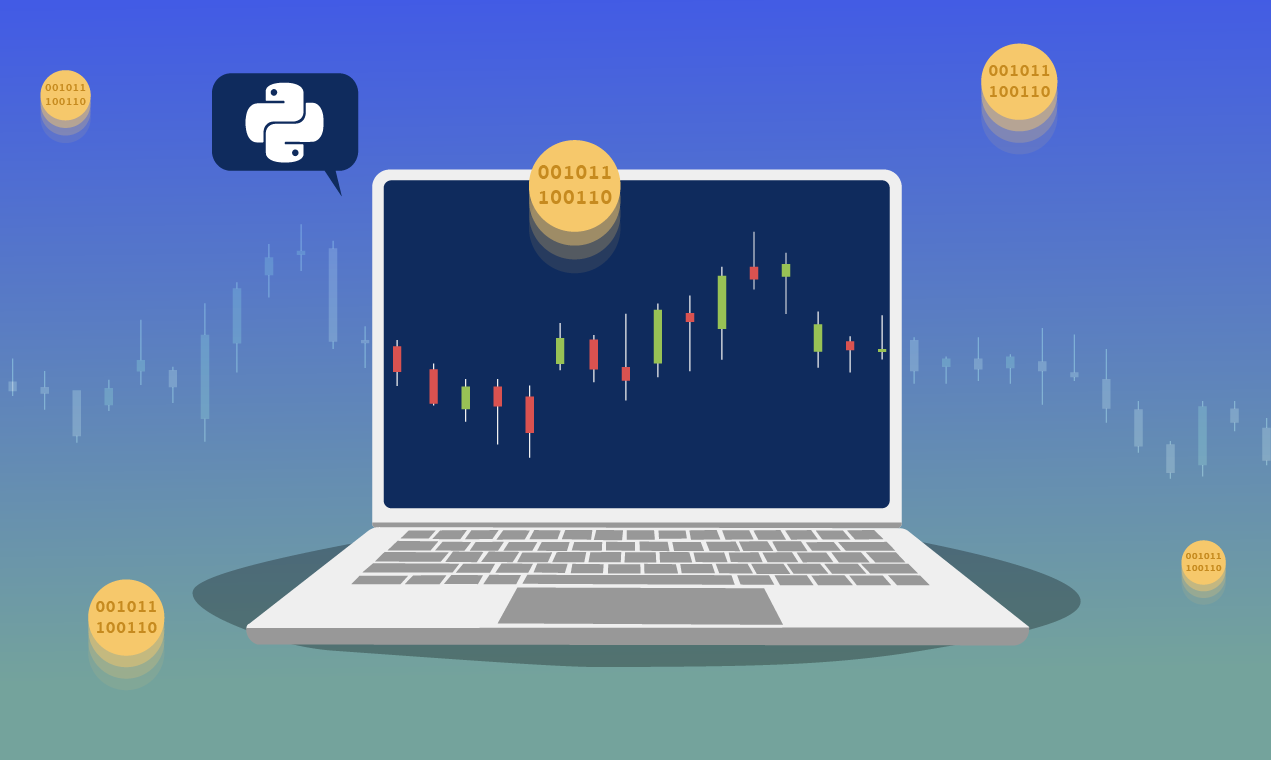
This article originally appeared on blog.blockmagnates, written by Craig Mariani
Please note that this article is for general informational purposes only. All screenshots are for illustrative purposes only. The views and opinions expressed are those of the author and do not reflect or represent the views and opinions of Alpaca. Alpaca does not recommend any specific securities, cryptocurrencies or investment strategies.
In this article we will discuss how to receive real time crypto data from an exchange using Python. Most retail algo traders use data that comes in batch format rather than real time; while this may get the job done for many algorithms involving day trading or swing trading, it’s too slow for algorithms that are faster such as high frequency trading algorithms. For this tutorial we will be using the Alpaca web sockets connection to stream this data, but this script will be able to work with other web sockets as well (you will need to modify the code for it to work properly though).

Install Dependencies
First we will need to install the libraries that we will be using. Open your command line and type the following commands (we will be installing the commands using pip):
$ pip install websocket
$ pip install alpaca-trade-api
$ pip install pprint
The websocket library in Python will be used for streaming our data and will be the bulk of our script, the alpaca-trade-api will be used to interact with our brokerage account, and pprint will be used to show the output of the data.
In order to interact with our broker we will also need to get our API keys. After signing up for an account, save these keys in a separate file. For this example we will be using the file secret.py
The Code
See the github link below to follow along:
We will need to import the libraries we just installed.
from websocket import create_connection
import simplejson as json
from secret import Secret
import pprint
Next we will need to create our connection to the websocket. The connection will be established with the websocket library and stored in the variable ws.
uri = 'wss://stream.data.alpaca.markets/v1beta1/crypto?exchanges=CBSE'
ws = create_connection(uri)
If the connection was a success you will get an output like below:
{'T': 'success', 'msg': 'connected'}
We now need to authenticate with the server which will be done with the code below. Notice how our API keys from Alpaca are being used in our json object in the places “Secret.key” and “Secret.secret_key”; both of these variables are imported from the “secret.py” file in the github repo. The connection we just made to the websocket will format the json object into a string and send it to the server.
auth_message = {"action":"auth","key": Secret.key, "secret": Secret.secret_key}
ws.send(json.dumps(auth_message))
We should get an output seen below:
{'T': 'success', 'msg': 'authenticated'}
We will then need to subscribe to the correct channel; in the example code below we will be subscribing to the latest trades of BTCUSD. (Alpaca also supports quotes and bar data across several different coins, but we will be focusing on trades of a single coin for this example.)
subscription = {"action":"subscribe","trades":["BTCUSD"]}
ws.send(json.dumps(subscription))
while True:
data = json.loads(ws.recv())
pprint.pprint(data[0])
We should get an output like this shown below:
{'T': 'subscription',
'bars': [],
'dailyBars': [],
'orderbooks': [],
'quotes': [],
'trades': ['BTCUSD'],
'updatedBars': []}
After receiving the correct message you should see the output data shown below:
{'S': 'BTCUSD',
'T': 't',
'i': 327851220,
'p': 34612.56,
's': 0.00080529,
't': '2022-05-08T04:23:58.158489Z',
'tks': 'S',
'x': 'CBSE'}
The keys (left side) of this Python dictionary describe different elements of the trade. Each of these elements are also described below:
“S” > the symbol for the trading pair or ticker we are looking at
“T” > the trade or the type of data we are looking at, if this were a bar the value for this key would be a “b”
“i” > the id of the trade. It can be used to identify different trades from each other
“p” > the latest price the asset was traded at
“s” > the size of the trade (quantity of asset) that were bought or sold
“t” > the time the trade was executed
“tks” > shows whether the asset was bought (B) or sold (S)
‘x’ > the exchange that the trade was executed on. Since this says “CBSE,” the trade was executed on the Coinbase exchange.
Notice that this is just one trade, when the script is executed you will be seeing multiple trades printing out on your terminal at a very fast pace.
About the Author
With an academic background in Computer Science, skills in Python, Linux, and MySQL, Craig Mariani works as a Data Scientist at NAVSEA Warfare Centers. Before working as a Data Scientist, he worked at his university as an Information Technology Technician.
Please note that this article is for informational purposes only. The example above is for illustrative purposes only. Actual crypto prices may vary depending on the market price at that particular time. Alpaca Crypto LLC does not recommend any specific cryptocurrencies.
Cryptocurrency is highly speculative in nature, involves a high degree of risks, such as volatile market price swings, market manipulation, flash crashes, and cybersecurity risks. Cryptocurrency is not regulated or is lightly regulated in most countries. Cryptocurrency trading can lead to large, immediate and permanent loss of financial value. You should have appropriate knowledge and experience before engaging in cryptocurrency trading. For additional information please click here.
Cryptocurrency services are made available by Alpaca Crypto LLC ("Alpaca Crypto"), a FinCEN registered money services business (NMLS # 2160858), and a wholly-owned subsidiary of AlpacaDB, Inc. Alpaca Crypto is not a member of SIPC or FINRA. Cryptocurrencies are not stocks and your cryptocurrency investments are not protected by either FDIC or SIPC. Please see the Disclosure Library for more information.
This is not an offer, solicitation of an offer, or advice to buy or sell cryptocurrencies, or open a cryptocurrency account in any jurisdiction where Alpaca Crypto is not registered or licensed, as applicable.