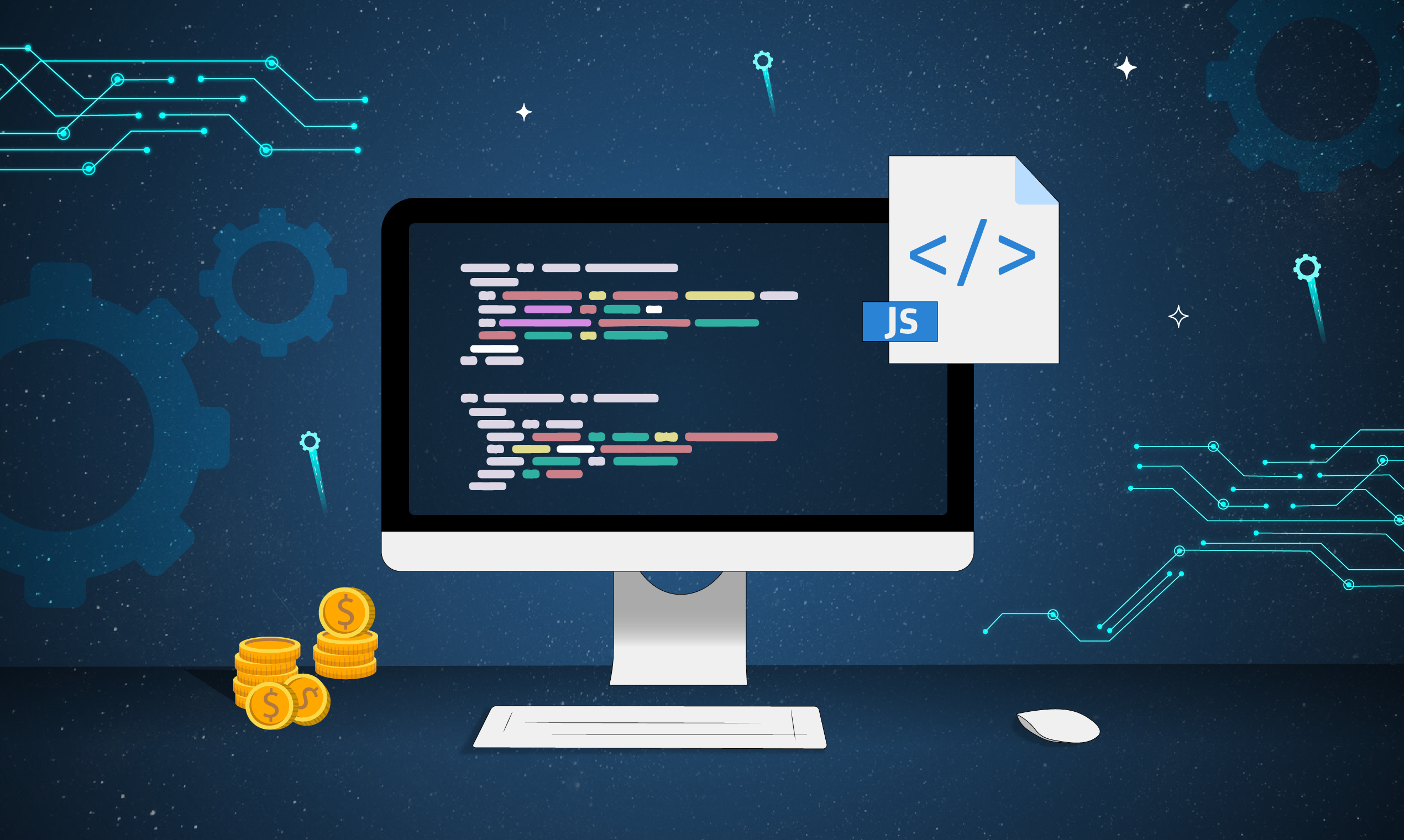
Please note that this article is for educational and informational purposes only. All screenshots are for illustrative purposes only. The views and opinions expressed are those of the author and do not reflect or represent the views and opinions of Alpaca. Alpaca does not recommend any specific securities or investment strategies.
This article originally appeared on Medium, written by Kendel Chopp
By the end of this tutorial, you should know how to make a simple request to the Alpaca API to retrieve your account information. I will be doing all of this with JavaScript and Node.js. Feel free to check out my repository on GitHub and the pull request associated with this tutorial. Also, if you are interested in a video version of this tutorial, feel free to check out my video on YouTube.
Install Node.js and npm
If you already have Node.js and npm feel free to skip this section. If not, I don’t plan on going in-depth as npm provides a great tutorial here.
Create a New Project
If you have used Node.js and npm before, you probably already know how to do this. If not, you will want to start by creating a directory to house the project. Alternatively, you can create a new remote repository somewhere (like GitHub or GitLab) and then clone that onto your computer. Once you have done that, enter your directory and type the following:
npm init
Once you have typed this, it will provide you with lots of prompts. Feel free to just click “enter” through them to take the defaults or enter options that better suit your needs. All of these can be changed later.
Install Alpaca Trading API
Next, we will use the Alpaca Trading API to make interacting with their web API even easier. You can check out the code for the npm package here on GitHub. To install the package, navigate to the same directory as your project and run:
npm install --save @alpacahq/alpaca-trade-api
After running this command, it should take a few seconds for everything to download, and then you should be all set up.
Sign Up for an Alpaca Account
You can sign up for an Alpaca account at https://alpaca.markets. If you just want to do paper trading you should only need to put in some very basic account information (only an email and password as of making this). If you would like to do some live trading, you will need some more information just like you would with any other brokerage.
Find Your API Keys
Once you have an Alpaca account, you will want to go to your account overview page. On the overview page, there should be a little section on the right-hand side that has keys or will let you generate some API keys. Remember, you need to keep these secret. If your keys are somehow leaked, be sure to reset them as soon as possible.
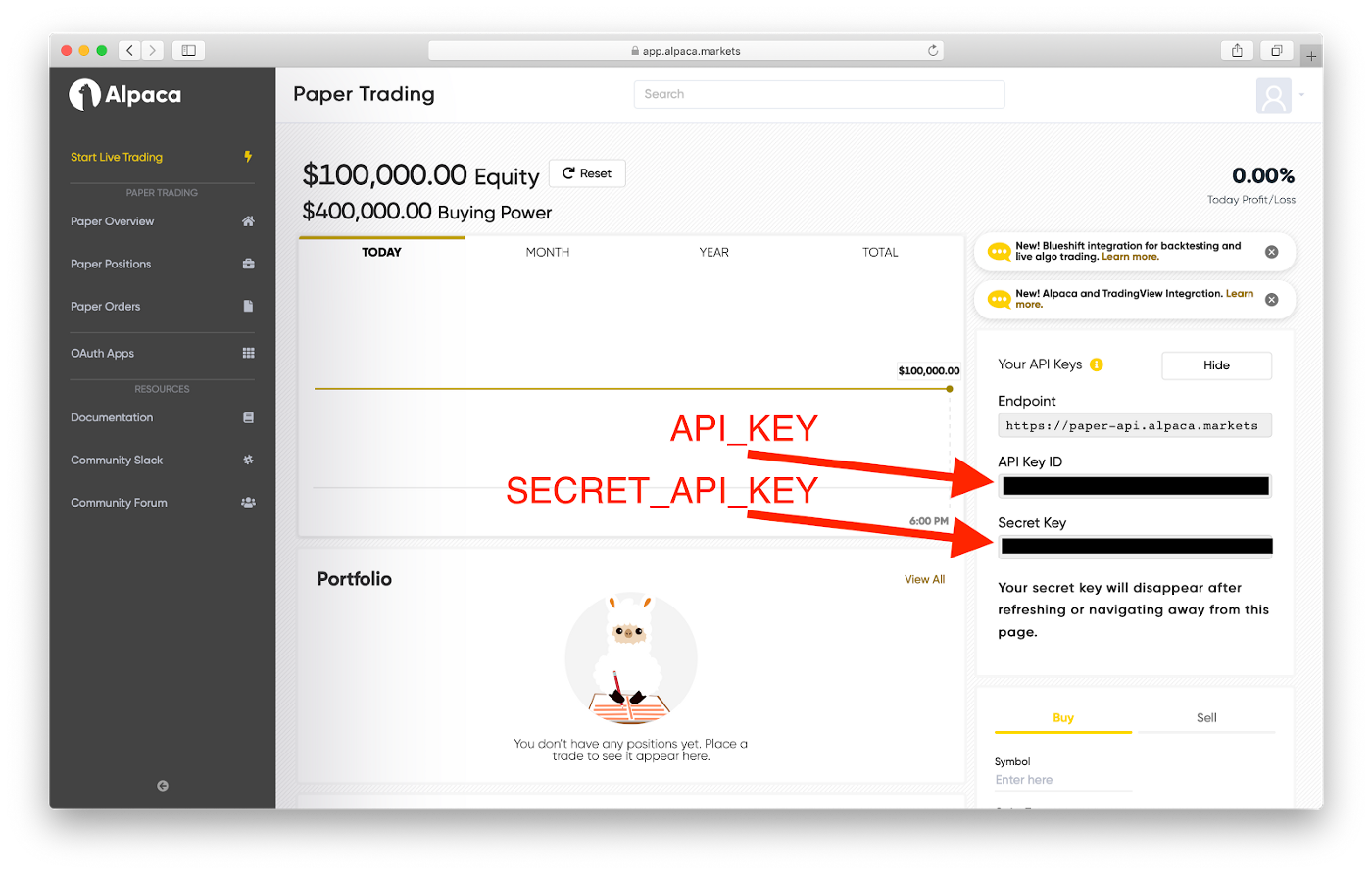
Once you can see the keys, stay on this page and head to the next step.
Create Environment Variables
In your project’s directory, create a new file called:
.env
Open this file up to edit, and we will add two environment variables to hold the API key and the secret API key. With the keys from the previous step, edit your environment file so it contains the following:
API_KEY=<insert your API key here>
SECRET_API_KEY=<insert your secret API key here>
Now, you can go ahead and close the Alpaca window with your keys since you have them. Additionally, if this is a git repository, you should put the .env
file into a .gitignore
so that you do not put your keys in your repository. To do this, simply run this command:
echo ‘.env’ > .gitignore
Now, the environment variable file will be ignored by git.
Create a Simple API Request
Now, we will begin coding. If you took the defaults when creating the npm project, you likely set the main file to index.js, so go ahead and create a file called index.js. Once you have this file, go ahead and open it in a text editor. Now, we will go line-by-line through the code. First, we need to import the Alpaca API package we installed earlier:
const Alpaca = require(‘@alpacahq/alpaca-trade-api’);
All that this line does is import everything we need from the Alpaca API package. Next, we need to create an alpaca object which will be how we access various API requests:
const alpaca = new Alpaca({
keyId: process.env.API_KEY,
secretKey: process.env.SECRET_API_KEY,
paper: true
});
There are lots of options we can use, but we will provide just the basic ones. First, our keyId
is the API_KEY
we got earlier, and the secretKey
is the SECRET_API_KEY
we got earlier from our account overview. In this case, I also set paper to true because I am doing paper trading, but you can, of course, set that to false for live trading.
Next, we can go ahead and create a simple asynchronous function to get your account info and log it to the console:
async function printAccount() {
const account = await alpaca.getAccount();
console.log(account);
}
We need this function to be asynchronous (see: async
) because alpaca.getAccount()
creates a network request (GET /account
) which we must wait to be fulfilled. In order to wait, we can use the await
keyword to wait for that request to finish. Once that request has finished, we log it to the console.
Lastly, we can go ahead and call the function we just wrote:
printAccount();
So, now we should have a file (index.js
) that looks something like this:
const Alpaca = require(‘@alpacahq/alpaca-trade-api’);
const alpaca = new Alpaca({
keyId: process.env.API_KEY,
secretKey: process.env.SECRET_API_KEY,
paper: true
});
async function printAccount() {
const account = await alpaca.getAccount();
console.log(account);
}
printAccount();
That is it for our code, and now we can move on to running this code.
Run Your Code
Now, we can go ahead and run our code! If you hop into that directory in a terminal window, we can simply run:
node index.js
This will run all of the code in our file. You should see something like the following:
{ id: ‘some-long-account-id-here’,
account_number: ‘some-account-number’,
status: ‘ACTIVE’,
currency: ‘USD’,
buying_power: ‘400000’,
regt_buying_power: ‘200000’,
daytrading_buying_power: ‘400000’,
cash: ‘100000’,
portfolio_value: ‘100000’,
pattern_day_trader: false,
trading_blocked: false,
transfers_blocked: false,
account_blocked: false,
created_at: ‘some-formatted-date-and-time’,
trade_suspended_by_user: false,
multiplier: ‘4’,
shorting_enabled: true,
equity: ‘100000’,
last_equity: ‘100000’,
long_market_value: ‘0’,
short_market_value: ‘0’,
initial_margin: ‘0’,
maintenance_margin: ‘0’,
last_maintenance_margin: ‘0’,
sma: ‘0’,
daytrade_count: 0 }
That’s it! You have now successfully run some code to use Alpaca’s API. You can read more into Alpaca’s documentation at https://alpaca.markets/docs/. You can also check out https://github.com/alpacahq/alpaca-trade-api-js for some more JavaScript examples. Obviously, this is only scratching the surface of algorithm trading, but it will get you up and running with the Alpaca API and open the door for so much more.
Once you are done with this, be sure to check out part two!

Alpaca does not prepare, edit, or endorse Third Party Content. Alpaca does not guarantee the accuracy, timeliness, completeness or usefulness of Third Party Content, and is not responsible or liable for any content, advertising, products, or other materials on or available from third party sites.
Brokerage services are provided by Alpaca Securities LLC ("Alpaca"), member FINRA/SIPC, a wholly-owned subsidiary of AlpacaDB, Inc. Technology and services are offered by AlpacaDB, Inc.
Interested in getting the latest news and content from Alpaca? Follow us on LinkedIn, Twitter, and Facebook for more.