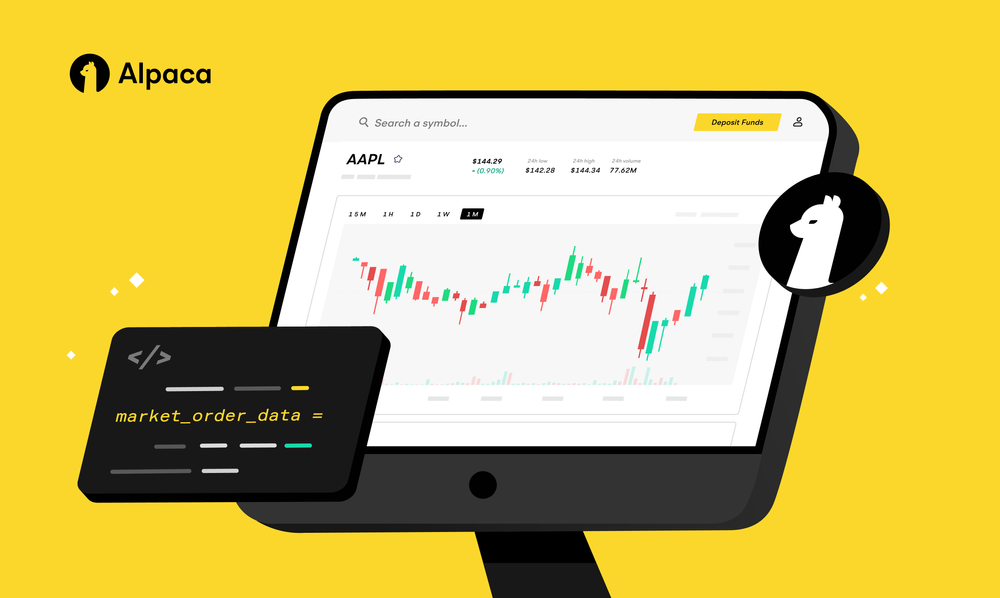
This blog was last updated on November 7, 2024.
Paper trading allows you to simulate trading without financial risk, providing a valuable way to test strategies and get familiar with Alpaca's Trading API. This guide walks you through the process.
Prerequisites
- Alpaca Account: Ensure you have an Alpaca Trading API account.
- Google Colab: Use your Google account to access Google Colab for running the necessary code.
Step 1: Fetch Your API Keys
- Log in to your Alpaca Trading API account. If you haven’t created an account yet, you can sign up or use our “How to Connect to Alpaca’s Trading API” as an onboarding guide.
- Obtain API Keys: Navigate to the API section to generate your API Keys. Note: It’s important to write down or save your Secret Key, as you will only have access to it once. If a new Secret Key is required, you can regenerate a new one. However, your current API Keys will be invalidated and you will need to re-establish your connection with your IDE.
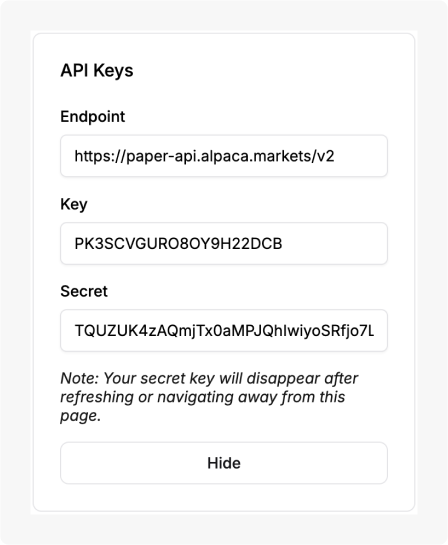
Step 2: Setting Up Google Colab
- Open Google Colab: Go to Google Colab in your browser.
- Create a new Notebook: Click the “New Notebook" button to initialize a Python environment.
- Install Required Packages: Install Alpaca-specific Python packages using:
!pip install alpaca-py requests
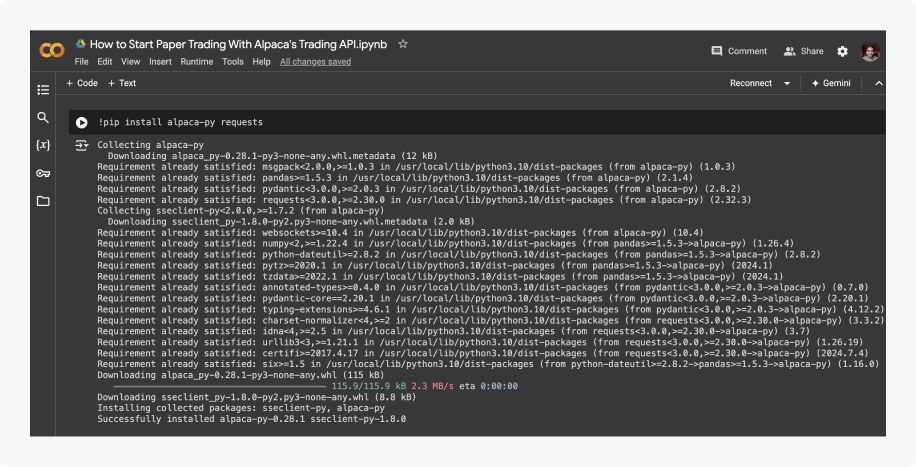
Step 3: Authentication and Connecting to Alpaca’s Trading API
- Connect to Alpaca’s Trading API: Initialize your connection using the following code. Replace '
YOUR_API_KEY_ID
' and 'YOUR_API_SECRET_KEY
' with your actual API Key and Secret Key, respectively.
import requests
from alpaca.trading.client import TradingClient
from alpaca.trading.requests import MarketOrderRequest
from alpaca.trading.enums import OrderSide, TimeInForce
trading_client = TradingClient('YOUR_API_KEY_ID', 'YOUR_API_SECRET_KEY', paper=True)
- Fetch Account Information: Verify your setup by fetching account details and using the following. Reminder to put your API Keys in their respective spots.
url = "https://paper-api.alpaca.markets/v2/account"
headers = {
"accept": "application/json",
"APCA-API-KEY-ID": "YOUR_API_KEY_ID",
"APCA-API-SECRET-KEY": "YOUR_API_SECRET_KEY"
}
response = requests.get(url, headers=headers)
print(response.text)
You should see your account details, confirming a successful connection and a paper trading environment.
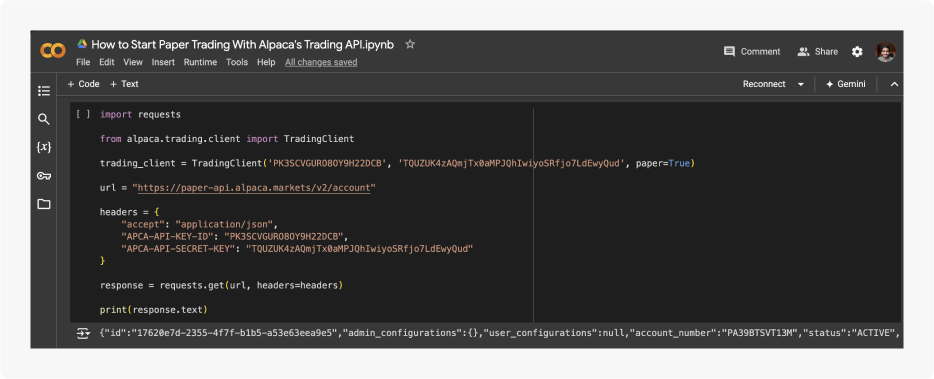
Step 4: Placing Paper Trades
Submit an Order: Use the API to submit a paper trading order. For example, to buy one share of Apple (AAPL):
# preparing market order
market_order_data = MarketOrderRequest(
symbol="AAPL",
qty=1,
side=OrderSide.BUY,
time_in_force=TimeInForce.DAY
)
# Market order
market_order = trading_client.submit_order(
order_data=market_order_data
)
Step 5: Check and Verify Your Paper Trades
Check Order Status: To make sure your paper order went through and to check the rest of your portfolio, use the following code:
# Get a list of all of our positions.
portfolio = trading_client.get_all_positions()
# Print the quantity of shares for each position.
for position in portfolio:
print("{} shares of {}".format(position.qty, position.symbol))
Verify Through Your Alpaca Dashboard: Alternatively, you can check your recent trades and portfolio in general by logging back into your Alpaca Trading Dashboard. Right from the homepage you’ll see your Top Positions and Recent Orders.
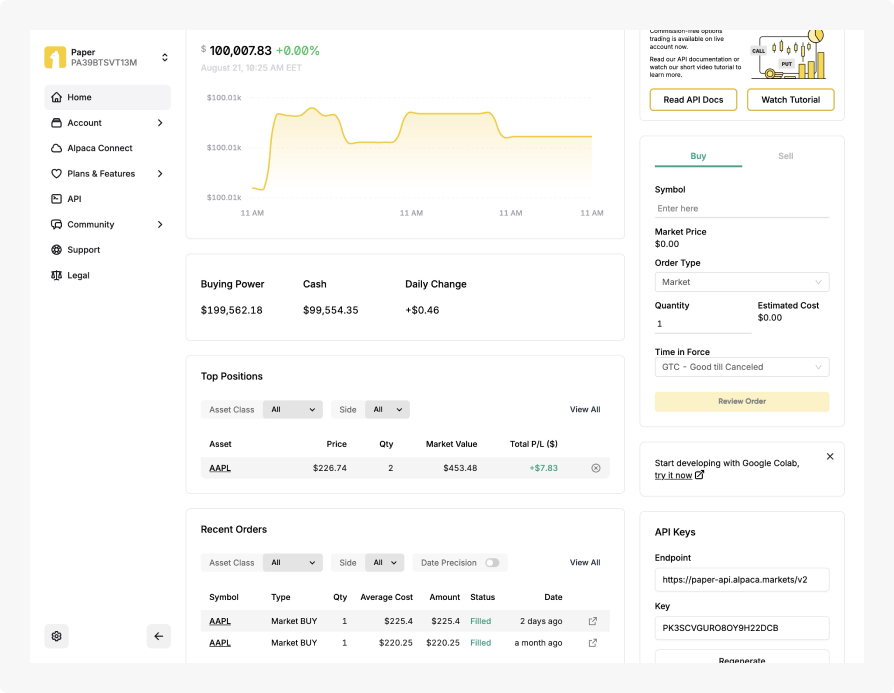
To see your full order history, click “View All” under Recent Orders, or under Account from the sidebar, click “Orders”.
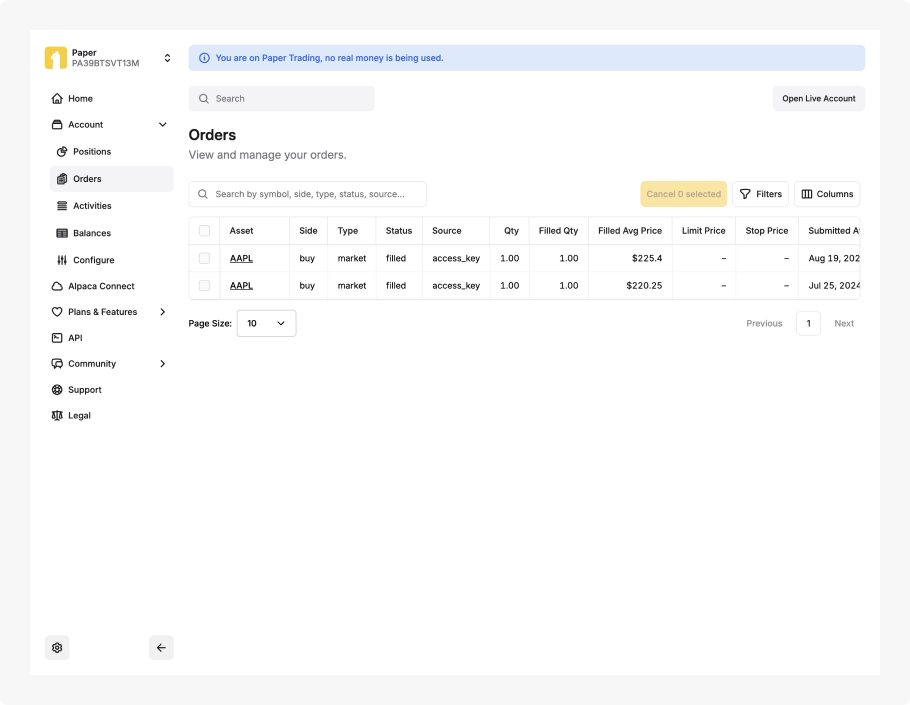
You are now ready to start paper trading with Alpaca's Trading API. This setup allows you to test your trading strategies risk-free.
For more advanced strategies and further reading, continue to explore Alpaca’s Trading API documentation and learn articles.
The Paper Trading API is offered by AlpacaDB, Inc. and does not require real money or permit a user to transact in real securities in the market. Providing use of the Paper Trading API is not an offer or solicitation to buy or sell securities, securities derivative or futures products of any kind, or any type of trading or investment advice, recommendation or strategy, given or in any manner endorsed by AlpacaDB, Inc. or any AlpacaDB, Inc. affiliate and the information made available through the Paper Trading API is not an offer or solicitation of any kind in any jurisdiction where AlpacaDB, Inc. or any AlpacaDB, Inc. affiliate (collectively, “Alpaca”) is not authorized to do business.
Please note that this article is for general informational purposes only and is believed to be accurate as of the posting date but may be subject to change. The examples above are for illustrative purposes only.
Securities brokerage services are provided by Alpaca Securities LLC ("Alpaca Securities"), member FINRA/SIPC, a wholly-owned subsidiary of AlpacaDB, Inc. Technology and services are offered by AlpacaDB, Inc.
This is not an offer, solicitation of an offer, or advice to buy or sell securities or open a brokerage account in any jurisdiction where Alpaca Securities are not registered or licensed, as applicable.