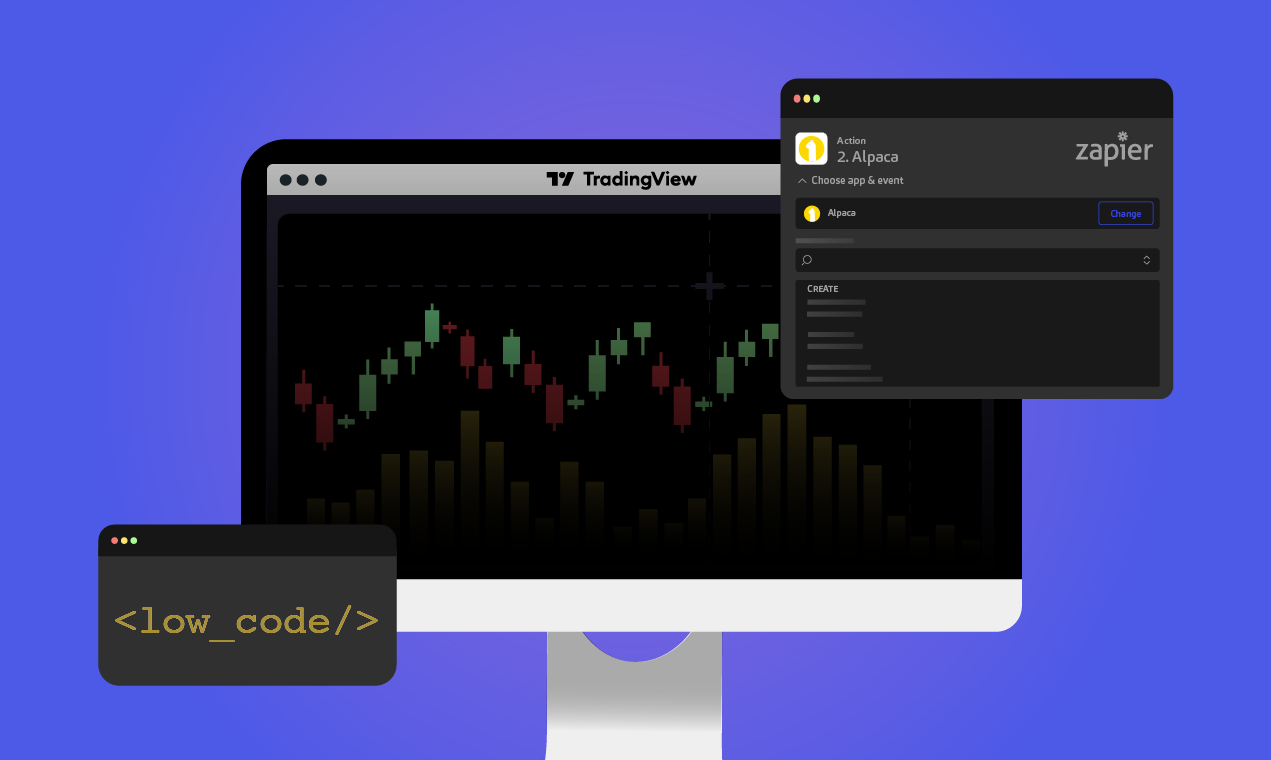
Alpaca and Zapier
In the previous article, we discussed how Zapier can be used to trigger trades on Alpaca. We went through a basic workflow that included a trigger from Twitter followed by trade execution on Alpaca.
That was pretty neat, but you know what’s better? Using a more sophisticated algo-trading strategy to trade on your behalf in under 15 lines of code! We will use Zapier’s low-code tooling to integrate a custom strategy with Alpaca’s trade execution.
What is TradingView?
TradingView is a charting platform that lets users create their own trading strategies, indicators and libraries. Users can then choose to share these with the community. It's a great platform to experiment and create your own algo-trading strategies.
We will use Pine script to write our custom strategy. This is a programming language developed by TradingView to write strategies and indicators. It's very similar to Python, so you can do your own technical analysis on TradingView using a Pine script if you have basic Python knowledge.
What are we building?
We're building a low-code workflow (Zap) that trades on our Alpaca account using a technical strategy that we will define. We will do this using a Pine script. This is one of the easiest ways to get started with Algo-trading and experimenting with different algorithms.
Steps involved in building this workflow are:
- Write a simple strategy using Pine script
- Setup Webhook alerts on TradingView
- Use conditional paths on Zapier to decide side of the trade on Alpaca
What do we need to get started?
You will need access to the following accounts to get started:
- Alpaca account
- Zapier Professional plan
- TradingView Pro plan
To begin trading using Alpaca, you need to create an account on Alpaca. You can sign up here. Although Zapier does offer a variety of free triggers and apps to work with, conditional paths require a Professional plan. Similarly, TradingView also needs to have a Pro plan enabled in order to use webhooks.
Let’s Build our Zap!
Algo-trading Strategy
A strategy is a Pine script that can send, modify and cancel buy/sell orders. Strategies allow you to perform backtesting (emulation of a strategy trading on historical data) and forward testing (emulation of a strategy trading on real-time data) according to your algorithms1.
We will create a very simple trading strategy based on moving averages. The idea is that whenever a short term moving average crosses above a long term moving average, it forms a chart pattern known as the Golden Cross. When the opposite happens, that is when the long term moving average crosses above the short term moving average it forms a chart pattern known as the Death Cross. Golden Cross is a bullish indicator while a Death Cross is a bearish indicator. We will use these indicators to buy and close our positions on Alpaca.
//@version=5
strategy("BTC SMA" ,overlay =true, initial_capital = 50, default_qty_type = strategy.percent_of_equity, default_qty_value = 100)
fast_ma_period = input(title = "Fast MA", defval = 5)
slow_ma_period = input(title = "Slow MA", defval =15)
fast_ma = ta.sma(close,fast_ma_period)
slow_ma = ta.sma(close,slow_ma_period)
plot(slow_ma, color = color.red, linewidth =1)
plot(fast_ma, color = color.blue, linewidth =2)
buy_condition = ta.crossover(fast_ma, slow_ma)
sell_condition = ta.crossover(slow_ma, fast_ma)
strategy.entry("buy_id", strategy.long, 0.001, when= buy_condition)
strategy.close("buy_id", when = sell_condition)
We begin by initializing our strategy using the strategy annotation call and name it “BTC SMA”. We pass it a few parameters apart from the name such as overlay, initial_capital, default_qty_type and default_qty_value. Overlay attribute takes in a boolean value that decides if the strategy plot will overlay the price chart. It is recommended that SMA strategies be overlaid on the price chart. We initialize an initial capital that we would like to backtest our strategy with. Default_qty_type and Default_qty_value then describe that we would like to use 100% of our capital to test this strategy.
Next, we are initializing a couple of inputs fast_ma_period
and slow_ma_period
. We are doing this so we can easily change the periods over which we would like to analyze the moving averages. We give it a title and a default value to get it initialized. The image below shows what it looks like on a TradingView chart.
Once we have the inputs initialized, we use the internal ta
library to use the sma
function. This function calculates the moving averages given a period and the bar prices for that chart. Here, we are using the closing prices of a bar to calculate the moving averages. Now we have our moving averages ready, we can plot them on the chart. We use the plot
function to accomplish this.
A strategy will also need conditional logic to understand when to place trades. The last 4 last lines of code are just about that. We define buy and sell conditions. Using ta
library’s ‘crossover’ function helps us define these. Buy_condition
occurs when a golden cross is formed and likewise a ‘sell_condition’ occurs when a death cross
is formed. Finally, we define our entry points based on the conditions we just defined. To define an entry point, we need to pass an id, direction of trade, quantity to trade and most importantly the condition when it needs to be bought. On the other hand, to define a closing point of our trade, we pass it the same id as our buy condition along with the condition that triggers the selling (sell_condition).
If you save this script in the pine editor you will see two SMA lines overlaying the BTC price chart.
Setting Webhook Alerts
Before we set up our webhook alerts on TradingView, we need to get the webhook url from Zapier that will receive the webhook requests.
We need to select Webhooks by Zapier
as our trigger application. Once the application is selected, it should prompt you to select an event type. Here, we are going to select "Catch a Raw Hook".
Once the event is selected and you press continue, you should see a unique webhook url. We need to copy this to the clipboard so we can use it to set up our TradingView alerts.
Next, we need to go back to our TradingView chart and click on the Alert icon on the top of the chart. This should bring up a pop up to create an Alert.
To create a webhook alert based on the strategy we just created, we need to select BTC SMA
as the condition. This means that whenever we enter a position or close it we should see an alert. We need to specify an expiration date for our alert too. You should keep this at least a few days apart from the date you are making this alert in case there is no change in technical trend.
Next, we make sure the Webhook URL
checkbox is ticked and paste the webhook url we copied earlier in the space provided as shown in the image below.
Finally, we create a message payload that Zapier is going to receive when this alert triggers. We can set this to a JSON object so it can be easily parsed. The message looks like this:
{
"side": {{strategy.order.action}}
}
Here, we are passing in a ‘side’ object with a value of ‘strategy.order.action’ which only takes two values: buy and sell. This means that if a buy condition is triggered on TradingView, Zapier is going to see a response that looks like this:
{
"side": buy}
}
Once we click on Create
, our webhook alert is ready to go!
Zapier needs to know what the webhook response looks like so we need to wait for the alert to trigger. A workaround for this wait is using Postman to send a dummy response that looks like what we have above. Keep in mind that this will need to be a POST request if you would like to mimic the TradingView response. Once an alert or a dummy response is sent, you can test the trigger on Zapier and should see a response similar to the one below.
Next, we need to create our conditional workflow so we can buy and sell when the condition arises.
Conditional Paths to execute trade on Alpaca
We have set our trigger, now it's time to set the action part of the workflow. To do this we start by selecting ‘Path’ from the list of applications. By default, ‘Path’ application gives us two conditional routes. We can add more if we like but in this case we just need 2 (1 for buying on Alpaca and 1 for selling).
We will edit Path A
to make it our Buy route. This means when we receive a webhook alert to buy BTC, we should execute this path.
To set this path, we need to give it a name. We have named it “Buy Route”. Once the name is set, we can define the rules for this conditional path. The rules are described in the following image.
You need to select Raw Body
in the Data dropdown field. Keep in mind that you will only see the option if an alert has been sent from TradingView or if you have made a dummy request using Postman.
Select contains
from the condition
dropdown box and enter buy in the text field on its right. Once these fields are set, your Buy route should look similar to the image below.
By clicking continue
, Zapier is going to test if the route would have executed given a webhook response (example data) we sent. If the dummy response or the webhook alert were successfully sent, you should see a screen similar to the one below.
Next, we will be prompted to select an action given the above condition is true. The condition defined above was to check if we received a buy response from the webhook. So for this action we will select Alpaca as the application and configure it to buy BTC for us.
Select the first option shown in the image above followed by Place Order
as the event. This is followed by a prompt that asks us to select an Alpaca account we would like to trade with. If you have connected your Alpaca account earlier, it should show up in the dropdown menu. If you haven’t done that already you will need to connect your account and authorize Alpaca to talk to Zapier. This process has also been described in a little more detail in [this](link here) article.
Once the account is authorized, we can configure our buy order. In the image below we are setting it up such that we buy $50 worth of BTCUSD on Alpaca.
Now that we have configured our Buy order, we can continue and test this action. Keep in mind that testing will place an actual order on your Alpaca account. You can skip this step if you do not want to place an action on Alpaca just yet.
We just created an entire workflow from scratch that lets you buy BTC on Alpaca in under 15 lines of code. That’s pretty cool!
Now, you need to do the same for the sell condition and set up a Sell Route as we did for the Buy Route.
The image below shows how the path rules for the Sell route should look like.
Once the Sell Route conditions are set, we can proceed and set the sell action on the Alpaca app. The set up should look similar to the one in the image below.
Hooray! We have finally configured our entire trading workflow and are ready to publish this Zap.
Takeaways
Zapier and TradingView are powerful tools that help you trade on Alpaca in a low-code way. Using these tools, we were able to successfully create our own trading strategy and send webhook alerts that triggered a trade action on Alpaca. One must remember to either wait for the TradingView app to send alerts to the webhook specified or mimic the response using Postman. This is because Zapier needs to know what kind of response it should expect before it triggers a conditional action on Alpaca. Also, keep in mind that the trading strategy used is used just for demonstration purposes and should be carefully evaluated when building your own trading setup.
Sources
Alpaca, Zapier Inc. and TradingView Inc. are not affiliated and none are responsible for the liabilities of the others.
There is no guarantee that any investment strategy will achieve its objectives.
Alpaca does not prepare, edit, or endorse Third Party Content. Alpaca does not guarantee the accuracy, timeliness, completeness or usefulness of Third Party Content, and is not responsible or liable for any content, advertising, products, or other materials on or available from third party sites.
Please note that this article is for general informational purposes only. All screenshots are for illustrative purposes only. The views and opinions expressed are those of the author and do not reflect or represent the views and opinions of Alpaca. Alpaca does not recommend any specific securities, cryptocurrencies or investment strategies.
All investments involve risk and the past performance of a security, or financial product does not guarantee future results or returns. Keep in mind that while diversification may help spread risk it does not assure a profit, or protect against loss. There is always the potential of losing money when you invest in securities, or other financial products. Investors should consider their investment objectives and risks carefully before investing.
Securities brokerage services are provided by Alpaca Securities LLC ("Alpaca Securities"), member FINRA/SIPC, a wholly-owned subsidiary of AlpacaDB, Inc. Technology and services are offered by AlpacaDB, Inc.
Cryptocurrency is highly speculative in nature, involves a high degree of risks, such as volatile market price swings, market manipulation, flash crashes, and cybersecurity risks. Cryptocurrency is not regulated or is lightly regulated in most countries. Cryptocurrency trading can lead to large, immediate and permanent loss of financial value. You should have appropriate knowledge and experience before engaging in cryptocurrency trading. For additional information please click here.
Cryptocurrency services are made available by Alpaca Crypto LLC ("Alpaca Crypto"), a FinCEN registered money services business (NMLS # 2160858), and a wholly-owned subsidiary of AlpacaDB, Inc. Alpaca Crypto is not a member of SIPC or FINRA. Cryptocurrencies are not stocks and your cryptocurrency investments are not protected by either FDIC or SIPC. Please see the Disclosure Library for more information.
This is not an offer, solicitation of an offer, or advice to buy or sell cryptocurrencies, or open a cryptocurrency account in any jurisdiction where Alpaca Crypto is not registered or licensed, as applicable.